python numpy三维数组排序
时间: 2023-07-20 21:22:05 浏览: 480
你可以使用 numpy 的 sort 函数进行三维数组排序。按照某个维度排序只需要设置 axis 参数即可。以下是一个示例代码,其中我们对一个 3x3x3 的三维数组按照第二维进行排序:
```python
import numpy as np
arr = np.array([[[9, 8, 7], [6, 5, 4], [3, 2, 1]],
[[12, 11, 10], [15, 14, 13], [18, 17, 16]],
[[21, 20, 19], [24, 23, 22], [27, 26, 25]]])
sorted_arr = np.sort(arr, axis=1)
print(sorted_arr)
```
输出结果:
```
[[[ 3 2 1]
[ 6 5 4]
[ 9 8 7]]
[[12 11 10]
[15 14 13]
[18 17 16]]
[[21 20 19]
[24 23 22]
[27 26 25]]]
```
如果你想按照其他维度排序,只需要改变 axis 参数即可。例如按照第三维排序:
```python
import numpy as np
arr = np.array([[[9, 8, 7], [6, 5, 4], [3, 2, 1]],
[[12, 11, 10], [15, 14, 13], [18, 17, 16]],
[[21, 20, 19], [24, 23, 22], [27, 26, 25]]])
sorted_arr = np.sort(arr, axis=2)
print(sorted_arr)
```
输出结果:
```
[[[ 1 2 3]
[ 4 5 6]
[ 7 8 9]]
[[10 11 12]
[13 14 15]
[16 17 18]]
[[19 20 21]
[22 23 24]
[25 26 27]]]
```
如果你想获取排序后的索引,可以使用 argsort 函数,例如:
```python
import numpy as np
arr = np.array([[[9, 8, 7], [6, 5, 4], [3, 2, 1]],
[[12, 11, 10], [15, 14, 13], [18, 17, 16]],
[[21, 20, 19], [24, 23, 22], [27, 26, 25]]])
sorted_indices = np.argsort(arr, axis=2)
print(sorted_indices)
```
输出结果:
```
[[[2 1 0]
[2 1 0]
[2 1 0]]
[[2 1 0]
[2 1 0]
[2 1 0]]
[[2 1 0]
[2 1 0]
[2 1 0]]]
```
阅读全文
相关推荐

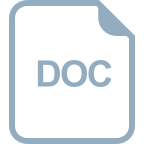
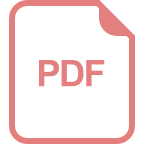

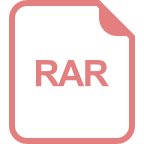
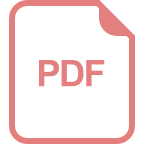
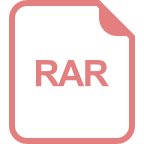
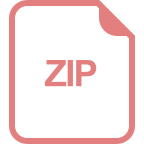
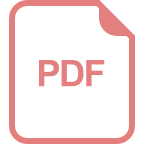
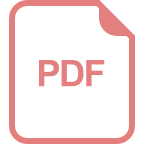
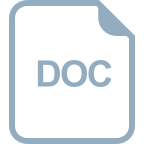
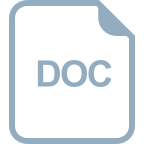
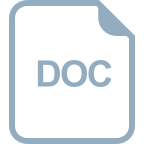
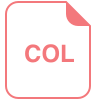
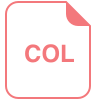
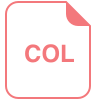