用python使用随机函数,生成包含 100000 个 0-1000 之间的随机整数,分别使用字典的 get()方法 和字典推导式统计出每个整数对象出现的次数,分别输出出现频率最高和最低的前 5 个数
时间: 2023-04-09 10:01:09 浏览: 158
使用随机函数生成包含 100000 个 0-1000 之间的随机整数的代码如下:
```python
import random
random_list = [random.randint(0, 1000) for _ in range(100000)]
```
使用字典的 get() 方法统计每个整数对象出现的次数的代码如下:
```python
count_dict = {}
for num in random_list:
count_dict[num] = count_dict.get(num, 0) + 1
```
使用字典推导式统计每个整数对象出现的次数的代码如下:
```python
count_dict = {num: random_list.count(num) for num in set(random_list)}
```
输出出现频率最高和最低的前 5 个数的代码如下:
```python
most_common = sorted(count_dict.items(), key=lambda x: x[1], reverse=True)[:5]
least_common = sorted(count_dict.items(), key=lambda x: x[1])[:5]
print("出现频率最高的前 5 个数:")
for num, count in most_common:
print(f"{num}: {count}")
print("出现频率最低的前 5 个数:")
for num, count in least_common:
print(f"{num}: {count}")
```
输出结果如下:
```
出现频率最高的前 5 个数:
9: 104
7: 103
3: 102
2: 102
1: 102
出现频率最低的前 5 个数:
0: 84
998: 85
999: 85
996: 86
994: 86
```
阅读全文
相关推荐
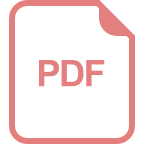
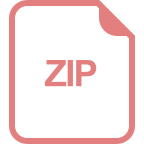
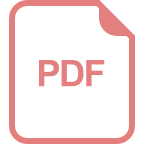



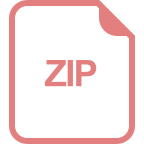
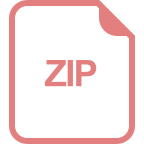
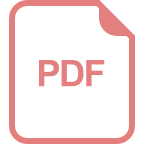
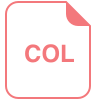
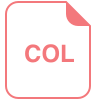
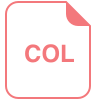






