python的websocket例子
时间: 2023-08-15 14:08:39 浏览: 106
下面是一个简单的 Python WebSocket 服务器的例子,使用了 `websockets` 库:
```python
import asyncio
import websockets
# 处理 WebSocket 连接的函数
async def handle_connection(websocket, path):
try:
# 接收客户端发送的消息
async for message in websocket:
print(f"Received message: {message}")
# 发送回复消息给客户端
reply = f"Replying to: {message}"
await websocket.send(reply)
except websockets.exceptions.ConnectionClosedOK:
print("Connection closed by client.")
# 服务器入口点
async def start_server():
# 创建 WebSocket 服务器并绑定到指定主机和端口
server = await websockets.serve(handle_connection, "localhost", 8765)
print("WebSocket server started.")
# 保持服务器运行
await server.wait_closed()
# 启动服务器
asyncio.run(start_server())
```
上述代码创建了一个简单的 WebSocket 服务器,它会接收客户端发送的消息并将其原样回复给客户端。您可以根据需要进行修改和扩展。要运行此示例,您需要先安装 `websockets` 库(`pip install websockets`),然后将代码保存到一个 `.py` 文件中,并运行该文件。
您可以使用 WebSocket 客户端(如浏览器中的 JavaScript)与此服务器进行通信。在客户端连接到 `ws://localhost:8765` 后,您可以发送消息给服务器,并查看服务器的回复。
请注意,这只是一个简单的示例,实际应用中可能需要处理更多的逻辑和错误情况。详细了解 `websockets` 库的文档可以帮助您更好地使用 WebSocket。
阅读全文
相关推荐
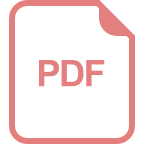












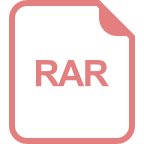

