近邻聚类算法 c++代码
时间: 2023-10-20 17:36:47 浏览: 98
以下是一个使用C语言实现的简单近邻聚类算法的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define NUM_POINTS 10
#define DIMENSIONS 2
#define CLUSTER_THRESHOLD 2.0
typedef struct {
double x;
double y;
} Point;
double get_distance(Point p1, Point p2) {
double dx = p2.x - p1.x;
double dy = p2.y - p1.y;
return sqrt(dx*dx + dy*dy);
}
int main() {
Point points[NUM_POINTS] = {{1, 2}, {3, 4}, {5, 6}, {7, 8}, {9, 10},
{11, 12}, {13, 14}, {15, 16}, {17, 18}, {19, 20}};
int cluster_id[NUM_POINTS]; int num_clusters = 0;
for (int i = 0; i < NUM_POINTS; i++) {
cluster_id[i] = -1;
}
for (int i = 0; i < NUM_POINTS; i++) {
if (cluster_id[i] == -1) {
cluster_id[i] = num_clusters;
num_clusters++;
for (int j = i+1; j < NUM_POINTS; j++) {
if (cluster_id[j] == -1) {
double distance = get_distance(points[i], points[j]);
if (distance <= CLUSTER_THRESHOLD) {
cluster_id[j] = cluster_id[i];
}
}
}
}
}
for (int i = 0; i < NUM_POINTS; i++) {
printf("Point (%f, %f) belongs to cluster %d\n", points[i].x, points[i].y, cluster_id[i]);
}
return 0;
}
```
这个代码示例中,我们定义了一个包含10个二维点的数组,然后使用近邻聚类算法将这些点进行聚类。首先,我们初始化每个点的聚类标识为-1,表示未被分配到任何聚类。然后,遍历每个点,如果该点未被分配到聚类,则将其作为新聚类的起始点,并将其聚类标识设置为当前聚类数。接下来,遍历剩余的未分配点,计算与当前起始点的距离,如果距离小于等于阈值,将其分配到同一聚类,并将其聚类标识设置为当前聚类数。最后,打印每个点所属的聚类。
请注意,这只是一个简单的示例,并且在实际应用中可能需要根据具体需求进行修改和优化。
阅读全文
相关推荐
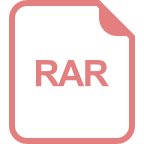

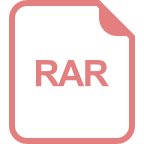
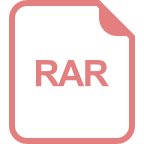
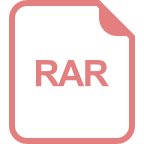
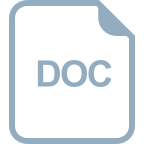
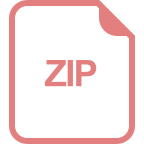
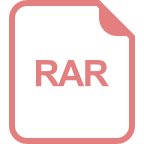
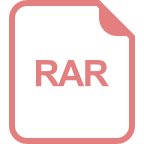
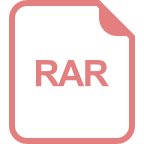
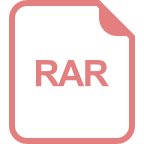
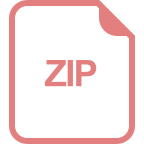
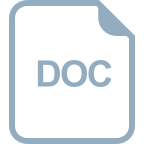
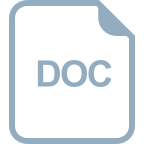
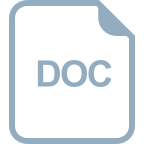