图像分割计算精确率代码
时间: 2023-12-10 09:03:23 浏览: 81
图像分割任务的精确率计算与分类任务略有不同。在图像分割中,我们通常使用像素级别的精确率来衡量模型的性能。以下是一个示例代码,用于计算图像分割任务的精确率:
```python
import numpy as np
def pixel_accuracy(y_true, y_pred):
# 将预测结果转换为二进制图像(0或1)
y_pred = np.round(y_pred).astype(np.uint8)
# 计算正确分类的像素数
correct_pixels = np.sum(y_true == y_pred)
# 计算总共的像素数
total_pixels = y_true.shape[0] * y_true.shape[1]
# 计算精确率
accuracy = correct_pixels / total_pixels
return accuracy
```
在这个示例代码中,`y_true` 是真实的分割图像,`y_pred` 是模型预测的分割图像。首先,我们将预测结果转换为二进制图像,这是因为通常模型输出为连续值。然后,我们计算正确分类的像素数,即真实标签和预测标签相等的像素数。最后,我们计算总共的像素数,并将正确分类的像素数除以总共的像素数,得到精确率的值。
请注意,这只是一个示例代码,具体实现可能会因任务和需求而有所不同。此代码假设输入的分割图像是二进制图像,如果你的场景中使用了多类别的分割任务,你可能需要进行适当的修改来计算每个类别的精确率。
相关问题
图像分割评估指标代码
图像分割评估指标是用来衡量图像分割算法性能的重要指标。以下是一个示例代码,用于计算图像分割的常见评估指标,包括精确度(Accuracy)、召回率(Recall)、特异度(Specificity)、F1分数(F1 Score)和IoU(Intersection over Union)。
```python
import numpy as np
def evaluate_segmentation(true_mask, pred_mask):
true_mask = true_mask.astype(bool)
pred_mask = pred_mask.astype(bool)
# 计算真阳性、假阳性和假阴性
true_positive = np.logical_and(true_mask, pred_mask).sum()
false_positive = np.logical_and(np.logical_not(true_mask), pred_mask).sum()
false_negative = np.logical_and(true_mask, np.logical_not(pred_mask)).sum()
# 计算精确度、召回率和特异度
accuracy = (true_positive + true_negative) / (true_positive + true_negative + false_positive + false_negative)
recall = true_positive / (true_positive + false_negative)
specificity = true_negative / (true_negative + false_positive)
# 计算F1分数
precision = true_positive / (true_positive + false_positive)
f1_score = 2 * (precision * recall) / (precision + recall)
# 计算IoU
intersection = np.logical_and(true_mask, pred_mask).sum()
union = np.logical_or(true_mask, pred_mask).sum()
iou = intersection / union
return accuracy, recall, specificity, f1_score, iou
# 示例用法
true_mask = np.array([[0, 1, 0],
[1, 1, 1],
[0, 1, 0]])
pred_mask = np.array([[0, 1, 0],
[0, 1, 0],
[0, 1, 0]])
accuracy, recall, specificity, f1_score, iou = evaluate_segmentation(true_mask, pred_mask)
print("Accuracy:", accuracy)
print("Recall:", recall)
print("Specificity:", specificity)
print("F1 Score:", f1_score)
print("IoU:", iou)
```
这段代码定义了一个`evaluate_segmentation`函数,该函数接受两个二值化的掩膜(true_mask和pred_mask),并计算出精确度、召回率、特异度、F1分数和IoU。在示例中,我们使用了一个3x3的真实掩膜和预测掩膜进行了计算,并打印了结果。
图像分割性能评价准确率的matlab代码
在MATLAB中,评估图像分割性能通常会使用准确率(Accuracy)、精确率(Precision)、召回率(Recall)和F1分数(F1 Score)等指标。这里是一个简单的示例,展示如何计算这些指标:
```matlab
% 假设我们有分割后的标签图(Ground Truth, GT)和预测结果(Predicted)
GT = ...; % 二值图像,0表示背景,1表示前景
Predicted = ...; % 同样的二值图像,预测的前景
% 首先将预测结果转换为与GT相同的格式
Predicted = imbinarize(Predicted);
% 计算像素级别的精度和召回率
true_positives = sum(GT(:) & Predicted(:)); % 正确识别的前景像素
false_positives = sum(Predicted(:) & ~GT(:)); % 错误地标记为前景的背景像素
false_negatives = sum(~GT(:) & ~Predicted(:)); % 错误地标记为背景的前景像素
true_negatives = sum(~GT(:) & Predicted(:)); % 正确识别的背景像素
% 计算准确率、精确率和召回率
accuracy = (true_positives + true_negatives) / numel(GT);
precision = true_positives / (true_positives + false_positives);
recall = true_positives / (true_positives + false_negatives);
% F1分数是精确率和召回率的调和平均值
f1_score = 2 * precision * recall / (precision + recall);
% 输出结果
fprintf('Accuracy: %.2f%%\n', accuracy*100);
fprintf('Precision: %.2f%%\n', precision*100);
fprintf('Recall: %.2f%%\n', recall*100);
fprintf('F1 Score: %.2f%%\n', f1_score*100);
% 如果需要,你可以将这些值保存到变量或结构体中,以便后续分析
results = struct('Accuracy', accuracy, 'Precision', precision, 'Recall', recall, 'F1Score', f1_score);
```
请注意,这只是一个基础示例,实际使用时可能需要根据具体的数据集和需求进行调整。此外,MATLAB中还有一些内置函数如`confusionmat`可以直接生成混淆矩阵,然后从中计算这些指标。
阅读全文
相关推荐
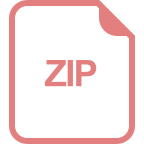
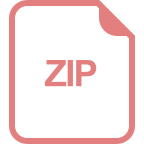
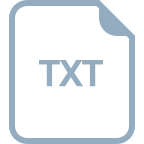
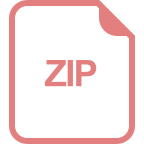
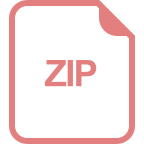
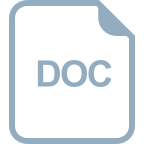
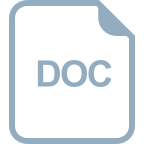
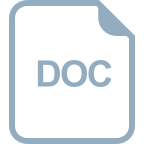
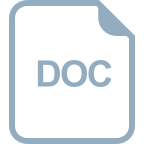
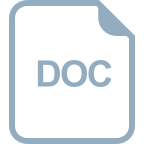
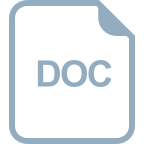
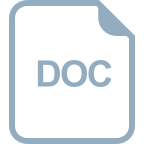
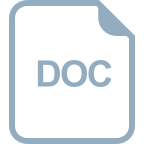
