使用c语言实现古典密码
时间: 2024-10-13 13:16:25 浏览: 33
在C语言中实现古典密码通常涉及到简单的替换或置换加密算法,比如凯撒密码。以下是凯撒密码的一个简单示例:
```c
#include <stdio.h>
#include <string.h>
// 函数:将输入字符串中的每个字符向右移动指定位数
char shift_char(char c, int s) {
if (c >= 'a' && c <= 'z') { // 对小写字母处理
return ((c - 'a' + s) % 26) + 'a';
} else if (c >= 'A' && c <= 'Z') { // 对大写字母处理
return ((c - 'A' + s) % 26) + 'A';
}
return c; // 对其他非字母字符不做处理
}
// 主函数:对整个字符串进行凯撒加密
void caesar_encrypt(char* plaintext, char* ciphertext, int shift) {
for (int i = 0; plaintext[i] != '\0'; i++) {
ciphertext[i] = shift_char(plaintext[i], shift);
}
ciphertext[i] = '\0'; // 确保字符串结束符也被替换
}
int main() {
char plaintext[100], ciphertext[100];
printf("请输入明文:");
fgets(plaintext, sizeof(plaintext), stdin); // 防止读入换行符
plaintext[strlen(plaintext)-1] = '\0'; // 去除fgets读取的换行符
int shift;
printf("请输入偏移量(1-25):");
scanf("%d", &shift);
caesar_encrypt(plaintext, ciphertext, shift);
printf("密文为:%s\n", ciphertext);
return 0;
}
```
在这个程序中,用户会被提示输入明文和加密偏移量(如3代表每个字母向后挪三位)。`caesar_encrypt`函数会遍历字符串并对每个字符进行替换。
阅读全文
相关推荐
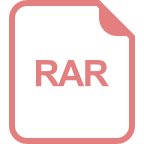
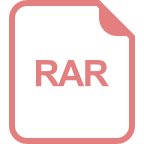

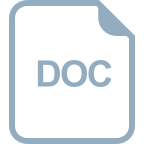
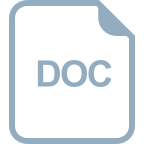
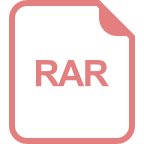
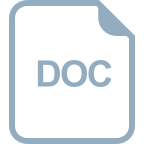
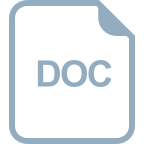
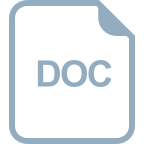
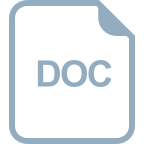
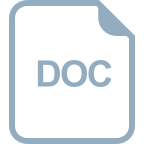
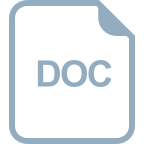




