vigenere密码c语言实现
时间: 2024-09-26 14:14:50 浏览: 68
Vigenère密码是一种古典加密技术,它通过将明文和密钥逐个字符相加并取模26来加密。在C语言中实现Vigenère密码,可以分为以下几个步骤:
1. 定义函数:创建一个函数,接受明文、密钥和加密长度作为参数。
2. 加密过程:遍历明文,对于每个字母,找到其对应在字母表中的位置(A=0,Z=25),然后加上密钥中对应位置的字母(同样按字母表计数)。如果结果超过25,就减去26以保持在字母范围内。
3. 转换回字符:获取加密后的字母在字母表的位置,将其转换回对应的字符。
4. 返回加密文本:将所有加密后的字符连接成字符串,返回给用户。
下面是一个简单的C语言示例代码片段:
```c
#include <stdio.h>
#include <string.h>
char encrypt_vigenere(char* plaintext, char* key, int len) {
const char alphabet[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int key_idx;
char encrypted_char;
for (int i = 0; i < len; ++i) {
if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
key_idx = (plaintext[i] - 'A' + key[i % strlen(key)] - 'A') % 26;
encrypted_char = alphabet[key_idx + 'A'];
} else if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
key_idx = (plaintext[i] - 'a' + key[i % strlen(key)] - 'a') % 26;
encrypted_char = alphabet[key_idx + 'a'];
} else {
encrypted_char = plaintext[i]; // 保留非字母字符不变
}
ciphertext[i] = encrypted_char;
}
ciphertext[len] = '\0'; // 结束符
return ciphertext;
}
void main() {
char plaintext[] = "HELLO WORLD";
char key[] = "KEY";
char* encrypted = encrypt_vigenere(plaintext, key, strlen(plaintext));
printf("Encrypted message: %s\n", encrypted);
free(encrypted); // 释放内存
}
```
阅读全文
相关推荐
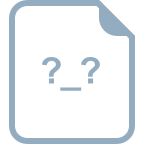
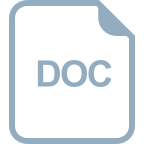
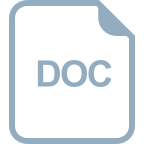
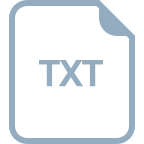
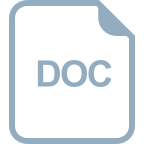



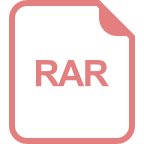
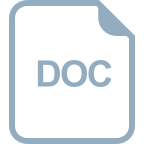








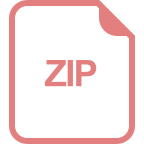