使用C语言实现多表代换密码中的Vigenere加密和解密方法
时间: 2024-09-27 21:06:59 浏览: 65
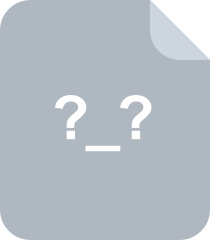
vigenere加密解密C语言代码
在C语言中实现Vigenère加密和解密方法,也称为维吉尼亚密码,通常涉及到两个步骤:密钥循环和替换字符。这是一种基于字母表移位的简单密码体制。
1. **加密** (Vigenère加密):
- **步骤1**: 获取明文和密钥字符串。对于每个字符,首先将明文字母转换为其ASCII码。
- **步骤2**: 将密钥的当前字母也转换为ASCII码,并根据字母表(通常为A-Z)找到其对应的数字索引。
- **步骤3**: 对明文字母的索引加上密钥字母的索引,然后取模26(因为字母表有26个字母)。得到的结果对应新的字母索引。
- **步骤4**: 根据新索引找到字母表中的新字符,这就是加密后的字符。如果结果超过25,需要从'A'重新开始计算。
- **步骤5**: 重复此过程,直到处理完整个明文。
2. **解密** (Vigenère解密):
- **步骤1**: 使用相同的密钥进行操作,但这次是在减法上做操作,即从新字符的索引减去密钥字母的索引,同样取模26。
- **步骤2**: 得到的结果对应原始字母索引,然后按照字母表找出对应的实际字母。
- **步骤3**: 继续这个过程直到处理完整个密文。
下面是一个简单的示例代码片段:
```c
#include <stdio.h>
#include <string.h>
char shift(char base, char key) {
return (base + (key % 26)) % 26 + 'A';
}
void encrypt(char *plaintext, char *key, char *ciphertext) {
int i = 0, j;
for (j = 0; plaintext[i] != '\0'; i++, j++) {
ciphertext[j] = shift(plaintext[i], key[i % strlen(key)]);
}
ciphertext[j] = '\0';
}
void decrypt(char *ciphertext, char *key, char *plaintext) {
encrypt(ciphertext, key, plaintext);
// 因为加密和解密实际上是互逆的,所以直接用上面的encrypt函数即可
}
int main() {
char plaintext[] = "HELLO WORLD";
char key[] = "KEY";
char ciphertext[100];
decrypt(ciphertext, key, plaintext); // 假设你需要解密
printf("Decrypted message: %s\n", ciphertext);
return 0;
}
```
阅读全文
相关推荐
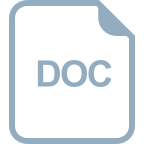















