编程实现多表代换密码中Vigenere加密和解密方法c语言
时间: 2024-09-27 07:07:27 浏览: 7
在C语言中,Vigenère加密是一种古典的多表替换式密码技术,它结合了一个简单的字母表替换(如凯撒密码)和一个固定长度的密钥循环。以下是基本的Vigenère加密和解密方法的实现:
### Vigenère加密
```c
#include <stdio.h>
#include <string.h>
// 函数声明
char vigenere_encrypt(char plaintext[], char key[], int length);
int main() {
char plaintext[] = "Hello, World!";
char key[] = "Key";
char ciphertext[ strlen(plaintext) + 1 ];
// 加密
strcpy(ciphertext, vigenere_encrypt(plaintext, key, strlen(key)));
printf("Encrypted text: %s\n", ciphertext);
return 0;
}
// Vigenère加密函数
char vigenere_encrypt(char plaintext[], char key[], int key_length) {
const char ALPHABET[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
char encrypted[26] = {0};
int i, j;
for (i = 0; plaintext[i]; ++i) {
if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
int shift = ALPHABET.find(key[j % key_length]);
encrypted[i] = ((plaintext[i] - 'A' + shift) % 26) + 'A';
j++;
} else if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
encrypted[i] = ((plaintext[i] - 'a' + ALPHABET.find(key[j % key_length])) % 26) + 'a';
j++;
} else {
encrypted[i] = plaintext[i];
}
}
return ciphertext;
}
```
### Vigenère解密
解密过程类似,只是将加法变成减法(移位方向相反),因为加密时是加上了密钥字符的偏移值。
```c
char vigenere_decrypt(char ciphertext[], char key[], int key_length) {
// 解密函数内容与加密函数相似,只需修改加法部分为减法
// ...
}
```
相关推荐
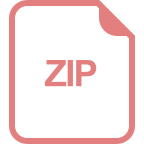















