编程实现多表代换密码中的Vigenere加密和解密方法
时间: 2024-09-27 16:12:26 浏览: 91
Vigenère加密是一种古典的表代换式密码,它通过将明文和一个称为“钥匙”的固定文本交替字符对齐来进行加密。下面是编程实现Vigenère加密和解密的基本步骤:
**加密 (Vigenère Cipher)**
1. **初始化**: 获取明文、钥匙和字母表(通常选择26个大写字母)。
2. **处理字符**: 将明文字母转换成小写并计算其相对于字母表的位置(索引从0开始)。同样,对钥匙的每个字符也做同样的操作。
3. **加密过程**: 对于每个明文字母,将它的位置加上钥匙当前位置的ASCII码值(减去'A'的ASCII码),然后取模26得到新的位置。这个新位置对应字母表上的一个字母就是加密后的结果。
4. **填充和替换**: 如果超过字母表范围,需要回绕到开头继续加,同时保留元音字母的大写。
**解密 (Vigenère Cipher Decryption)**
1. **重复上述加密过程,但是使用相反的钥匙(即原钥匙的逆序)进行计算**。这会抵消每次加密的增量,恢复出原始明文。
以下是简单的Python示例代码片段(假设使用大写字母):
```python
def encrypt(plaintext, key):
alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
ciphertext = ''
for i in range(len(plaintext)):
plaintext_char = plaintext[i]
key_char = key[i % len(key)] # 循环使用钥匙
index_plaintext = alphabet.index(plaintext_char)
index_key = alphabet.index(key_char)
encrypted_index = (index_plaintext + index_key) % 26
ciphertext += alphabet[encrypted_index]
return ciphertext
def decrypt(ciphertext, key):
return encrypt(ciphertext, key)[::-1] # 使用逆序钥匙解密
# 示例
plaintext = "HELLO WORLD"
key = "KEY"
ciphertext = encrypt(plaintext, key)
decrypted_text = decrypt(ciphertext, key)
```
阅读全文
相关推荐
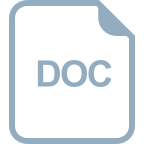
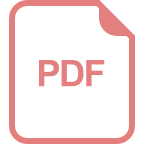
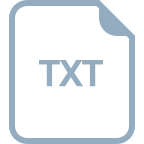




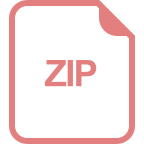
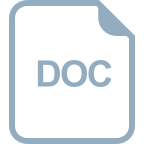
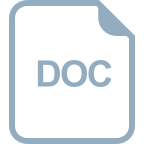
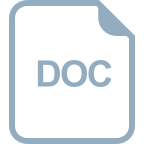
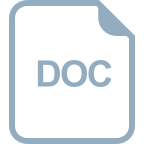
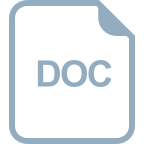
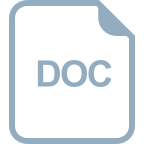
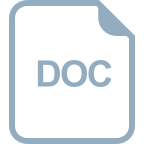
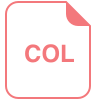


