c语言实现多表代换密码中Vigenere 加密和解密方法
时间: 2024-09-27 16:06:41 浏览: 42
在C语言中,Vigenère加密和解密方法通常用于实施多表代换密码(也称为Polyalphabetic Substitution Cipher),这是一种古典密码体制,通过多次应用简单的字母替换来隐藏信息。Vigenère密码使用了一个固定的关键词(key)对明文进行加密。
**加密过程(Vigenère加密)**:
1. **设置工作表**: 创建一个包含26个字母的加密表,其中每个字母对应另一个字母,通常是按照Caesar cipher的方式(每个字母向后移动固定位数)。关键词长度决定加密表的周期。
2. **循环处理**: 对于每个明文字母,首先找到关键词当前对应的字母(按字母顺序),然后在这个加密表中找到这个位置的字母作为密文字母。如果超过字母表范围,就从开头开始重置。
3. **重复关键词**: 如果明文比关键词长,需要将关键词重复直到其长度与明文相等。
**解密过程(Vigenère解密)**:
1. **逆向操作**: 使用相同的关键词和加密表,但是这次查找的是密文字母在表中的原位置,即它对应于哪个字母。
2. **还原字母**: 每次找到的原位置字母就是解密后的明文字符。
**示例代码片段(简化版)**:
```c
#include <stdio.h>
#include <string.h>
char encrypt(char plain, char key) {
int shift = (key - 'A') % 26;
return ((plain + shift - 'A') % 26) + 'A';
}
void vigenere_encrypt(char *plaintext, const char *keyword, char *ciphertext) {
for (int i = 0; plaintext[i]; ++i) {
ciphertext[i] = encrypt(plaintext[i], keyword[i % strlen(keyword)]);
}
}
// 解密函数类似,只需改变加密算法的部分
// ...
int main() {
char plaintext[] = "HELLO WORLD";
char keyword[] = "KEY";
char ciphertext[strlen(plaintext) + strlen(keyword)];
vigenere_encrypt(plaintext, keyword, ciphertext);
printf("Encrypted: %s\n", ciphertext);
// 解密部分省略...
return 0;
}
```
阅读全文
相关推荐
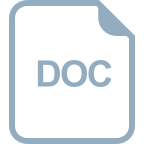




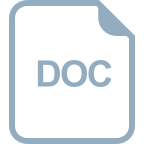
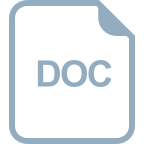
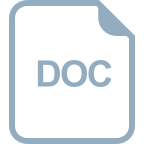






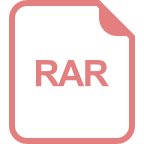
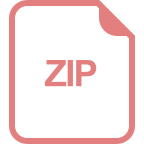