使用C语言实现仿射密码和Vigenere密码
时间: 2024-05-08 11:17:51 浏览: 15
仿射密码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
int inverse(int a, int m) {
a = a % m;
for (int x = 1; x < m; x++) {
if ((a * x) % m == 1) {
return x;
}
}
}
void affine_encrypt(char* plaintext, int key_a, int key_b) {
int len = strlen(plaintext);
for (int i = 0; i < len; i++) {
if (isalpha(plaintext[i])) {
plaintext[i] = toupper(plaintext[i]);
plaintext[i] = ((key_a * (plaintext[i] - 'A') + key_b) % 26) + 'A';
}
}
}
void affine_decrypt(char* ciphertext, int key_a, int key_b) {
int len = strlen(ciphertext);
int a_inverse = inverse(key_a, 26);
for (int i = 0; i < len; i++) {
if (isalpha(ciphertext[i])) {
ciphertext[i] = toupper(ciphertext[i]);
ciphertext[i] = (a_inverse * (ciphertext[i] - 'A' - key_b + 26)) % 26 + 'A';
}
}
}
int main() {
char plaintext[] = "HELLO WORLD";
int key_a = 5;
int key_b = 8;
printf("Plaintext: %s\n", plaintext);
affine_encrypt(plaintext, key_a, key_b);
printf("Encrypted Text: %s\n", plaintext);
affine_decrypt(plaintext, key_a, key_b);
printf("Decrypted Text: %s\n", plaintext);
return 0;
}
```
Vigenere密码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void vigenere_encrypt(char* plaintext, char* key) {
int len = strlen(plaintext);
int key_len = strlen(key);
for (int i = 0, j = 0; i < len; i++, j++) {
if (j == key_len) {
j = 0;
}
if (isalpha(plaintext[i])) {
plaintext[i] = toupper(plaintext[i]);
key[j] = toupper(key[j]);
plaintext[i] = ((plaintext[i] - 'A') + (key[j] - 'A')) % 26 + 'A';
}
}
}
void vigenere_decrypt(char* ciphertext, char* key) {
int len = strlen(ciphertext);
int key_len = strlen(key);
for (int i = 0, j = 0; i < len; i++, j++) {
if (j == key_len) {
j = 0;
}
if (isalpha(ciphertext[i])) {
ciphertext[i] = toupper(ciphertext[i]);
key[j] = toupper(key[j]);
ciphertext[i] = ((ciphertext[i] - 'A') - (key[j] - 'A') + 26) % 26 + 'A';
}
}
}
int main() {
char plaintext[] = "HELLO WORLD";
char key[] = "KEY";
printf("Plaintext: %s\n", plaintext);
vigenere_encrypt(plaintext, key);
printf("Encrypted Text: %s\n", plaintext);
vigenere_decrypt(plaintext, key);
printf("Decrypted Text: %s\n", plaintext);
return 0;
}
```
相关推荐
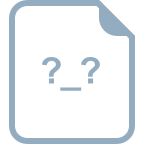















