用c语言编程实现仿射密码
时间: 2023-10-09 22:14:18 浏览: 182
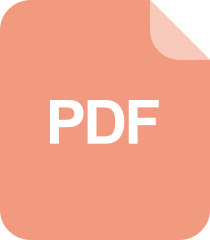
Python实现仿射密码

实现仿射密码需要以下步骤:
1. 定义仿射密码的加密算法和解密算法;
2. 实现输入明文和密钥,输出密文的加密函数;
3. 实现输入密文和密钥,输出明文的解密函数;
4. 对于输入的明文或密文,需要进行字符集转换。
下面是一个简单的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define ALPHABET_SIZE 26
// 加密函数
char* affine_encrypt(char* plaintext, int a, int b) {
char* ciphertext = (char*) malloc(sizeof(char));
int i;
for (i = 0; plaintext[i] != '\0'; i++) {
if (isalpha(plaintext[i])) {
char c = plaintext[i];
if (isupper(c)) {
ciphertext[i] = ((a * (c - 'A') + b) % ALPHABET_SIZE) + 'A';
} else {
ciphertext[i] = ((a * (c - 'a') + b) % ALPHABET_SIZE) + 'a';
}
} else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[i] = '\0';
return ciphertext;
}
// 解密函数
char* affine_decrypt(char* ciphertext, int a, int b) {
char* plaintext = (char*) malloc(sizeof(char));
int i, a_inverse;
for (a_inverse = 0; a_inverse < ALPHABET_SIZE; a_inverse++) {
if ((a * a_inverse) % ALPHABET_SIZE == 1) {
break;
}
}
for (i = 0; ciphertext[i] != '\0'; i++) {
if (isalpha(ciphertext[i])) {
char c = ciphertext[i];
if (isupper(c)) {
plaintext[i] = ((a_inverse * (c - 'A' - b + ALPHABET_SIZE)) % ALPHABET_SIZE) + 'A';
} else {
plaintext[i] = ((a_inverse * (c - 'a' - b + ALPHABET_SIZE)) % ALPHABET_SIZE) + 'a';
}
} else {
plaintext[i] = ciphertext[i];
}
}
plaintext[i] = '\0';
return plaintext;
}
int main() {
char plaintext[100], *ciphertext, *decryptedtext;
int a, b;
printf("Enter the plaintext: ");
scanf("%[^\n]s", plaintext);
printf("Enter the values of a and b: ");
scanf("%d %d", &a, &b);
// 加密
ciphertext = affine_encrypt(plaintext, a, b);
printf("Encrypted text: %s\n", ciphertext);
// 解密
decryptedtext = affine_decrypt(ciphertext, a, b);
printf("Decrypted text: %s\n", decryptedtext);
free(ciphertext);
free(decryptedtext);
return 0;
}
```
其中,`affine_encrypt()` 函数接受一个字符串明文和两个整数 `a` 和 `b` 作为输入,输出一个字符串密文。`affine_decrypt()` 函数接受一个字符串密文和两个整数 `a` 和 `b` 作为输入,输出一个字符串明文。在解密函数中,使用扩展欧几里得算法求出 `a` 的逆元素 `a_inverse`,然后计算出明文字符。
在主函数中,输入明文和密钥,然后调用加密和解密函数进行测试。注意,由于 C 语言中字符串必须以空字符结尾,因此在输出字符串时需要在末尾添加一个空字符。同时,由于使用了动态内存分配,需要在程序结束时释放分配的内存。
阅读全文
相关推荐

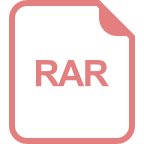
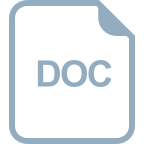
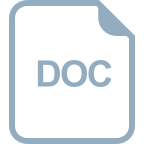



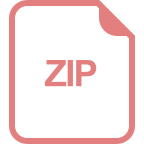
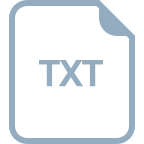
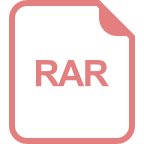
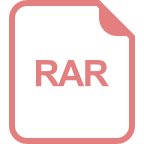
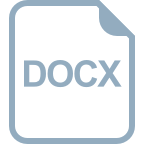
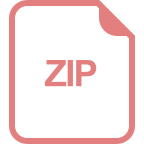
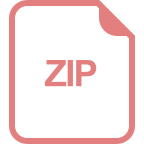
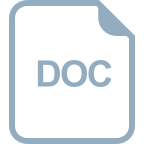
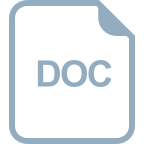