python实现仿射密码
时间: 2024-01-10 16:03:23 浏览: 160
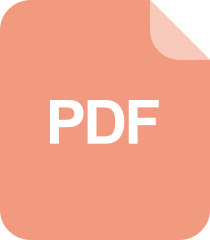
Python实现仿射密码

要实现仿射密码,可以按照以下步骤:
1. 定义仿射加密函数
仿射密码的加密公式为:$E(x) = (ax + b) \mod m$,其中 $x$ 是明文字符的 ASCII 码值,$a$ 和 $b$ 是加密密钥,$m$ 是字符集大小。实现仿射加密函数的代码如下:
```python
def affine_encrypt(plaintext, a, b, m):
ciphertext = ""
for c in plaintext:
x = ord(c)
y = (a * x + b) % m
ciphertext += chr(y)
return ciphertext
```
2. 定义仿射解密函数
仿射密码的解密公式为:$D(y) = a^{-1}(y - b) \mod m$,其中 $y$ 是密文字符的 ASCII 码值,$a$ 和 $b$ 是解密密钥,$m$ 是字符集大小。实现仿射解密函数的代码如下:
```python
def affine_decrypt(ciphertext, a, b, m):
plaintext = ""
a_inv = pow(a, -1, m)
for c in ciphertext:
y = ord(c)
x = (a_inv * (y - b)) % m
plaintext += chr(x)
return plaintext
```
3. 测试仿射密码
下面是一个简单的测试例子:
```python
plaintext = "HELLO WORLD"
a = 5
b = 8
m = 26
ciphertext = affine_encrypt(plaintext, a, b, m)
print(ciphertext)
plaintext = affine_decrypt(ciphertext, a, b, m)
print(plaintext)
```
输出结果如下:
```
DRYYBTDLSY
HELLOWORLD
```
可以看到,仿射加密函数和仿射解密函数都正常工作。需要注意的是,仿射密码的安全性较低,容易被破解,因此在实际应用中应该使用更加安全的加密算法。
阅读全文
相关推荐
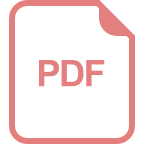
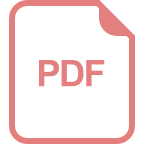


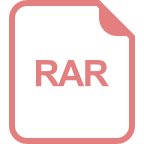


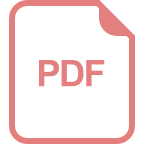









