仿射密码python代码实现,模数为26
时间: 2024-09-11 07:04:06 浏览: 63
仿射密码是一种简单的替换加密技术,属于经典的密码学算法之一。在仿射密码中,每个字母在密文中都由另一个字母替代,这个替代关系通过一个线性函数来定义。当我们设定模数为26时,实际上是在处理英文的26个字母。仿射加密可以使用以下公式来表示:
C = (a * P + b) mod 26
其中,C是密文字符,P是明文字符,a和b是密钥,且a和26互质,以保证每个明文字符都有一个唯一的密文字符相对应。
下面是一个简单的Python代码实现仿射密码加密和解密的例子:
```python
def egcd(a, b):
if a == 0:
return (b, 0, 1)
else:
g, y, x = egcd(b % a, a)
return (g, x - (b // a) * y, y)
def modinv(a, m):
g, x, y = egcd(a, m)
if g != 1:
raise Exception('Modular inverse does not exist')
else:
return x % m
def affine_encrypt(plain_text, a, b):
encrypted_text = ""
for char in plain_text:
if char.isalpha():
shift = ord(char.lower()) - ord('a')
new_shift = (a * shift + b) % 26
if char.isupper():
encrypted_text += chr(new_shift + ord('A'))
else:
encrypted_text += chr(new_shift + ord('a'))
else:
encrypted_text += char
return encrypted_text
def affine_decrypt(encrypted_text, a, b):
a_inv = modinv(a, 26)
decrypted_text = ""
for char in encrypted_text:
if char.isalpha():
shift = ord(char.lower()) - ord('a')
new_shift = (a_inv * (shift - b)) % 26
if char.isupper():
decrypted_text += chr(new_shift + ord('A'))
else:
decrypted_text += chr(new_shift + ord('a'))
else:
decrypted_text += char
return decrypted_text
# 使用示例
plain_text = "HELLO WORLD"
a = 7 # 选择a和26互质
b = 3 # 密钥b
encrypted = affine_encrypt(plain_text, a, b)
decrypted = affine_decrypt(encrypted, a, b)
print("原文: ", plain_text)
print("密文: ", encrypted)
print("解密: ", decrypted)
```
在这个实现中,我们首先定义了扩展欧几里得算法`egcd`和模逆元计算函数`modinv`,这是因为我们需要找到a关于26的模逆元来完成解密过程。接着,我们定义了加密函数`affine_encrypt`和解密函数`affine_decrypt`,分别实现了仿射密码的加密和解密过程。
阅读全文
相关推荐
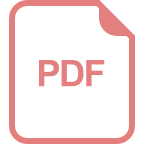
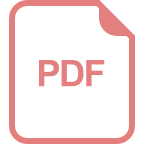
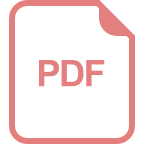



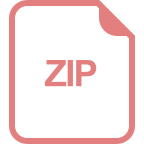
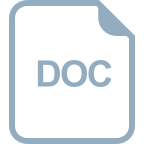
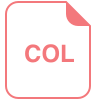


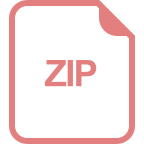
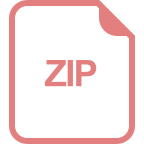
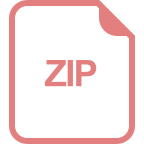
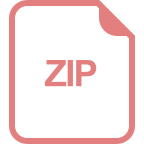
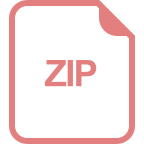
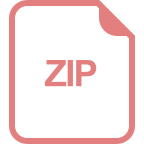
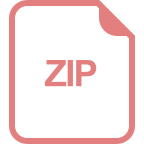
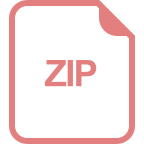