用c语言实现密码学仿射变换
时间: 2023-10-26 10:05:32 浏览: 152
密码学仿射变换是一种简单的加密算法,可以用来加密和解密文本。它基于线性变换,将每个字母用另一个字母替代。
以下是用C语言实现密码学仿射变换的示例代码:
```c
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
// 仿射变换加密函数
void affine_encrypt(char* plaintext, int a, int b) {
int len = strlen(plaintext);
char ciphertext[len];
for(int i=0; i<len; i++) {
// 将大写字母转换为数字
int x = plaintext[i] - 'A';
// 进行仿射变换
x = (a*x + b) % 26;
// 将数字转换为大写字母
ciphertext[i] = x + 'A';
}
// 打印加密后的密文
printf("Cipher text: %s\n", ciphertext);
}
// 仿射变换解密函数
void affine_decrypt(char* ciphertext, int a, int b) {
int len = strlen(ciphertext);
char plaintext[len];
// 计算a的逆元,用于解密
int a_inv = 0;
for(int i=0; i<26; i++) {
if((a*i) % 26 == 1) {
a_inv = i;
break;
}
}
for(int i=0; i<len; i++) {
// 将大写字母转换为数字
int x = ciphertext[i] - 'A';
// 进行仿射变换的逆操作
x = (a_inv * (x - b + 26)) % 26;
// 将数字转换为大写字母
plaintext[i] = x + 'A';
}
// 打印解密后的明文
printf("Plain text: %s\n", plaintext);
}
int main() {
char plaintext[100];
int a, b;
// 输入明文,a和b的值
printf("Enter plain text: ");
scanf("%s", plaintext);
printf("Enter value of a: ");
scanf("%d", &a);
printf("Enter value of b: ");
scanf("%d", &b);
// 加密明文
affine_encrypt(plaintext, a, b);
// 解密密文
char ciphertext[100];
strcpy(ciphertext, "ZLKVWFY");
affine_decrypt(ciphertext, a, b);
return 0;
}
```
在上面的示例代码中,我们定义了两个函数affine_encrypt()和affine_decrypt(),分别用于加密和解密文本。其中,a和b是仿射变换的参数,plaintext是明文,ciphertext是密文。
在主函数中,我们首先输入明文和a、b的值,然后调用affine_encrypt()函数进行加密,最后调用affine_decrypt()函数进行解密。在这里,我们手动指定了一个密文,你可以根据需要修改。
注意,由于这是一个简单的加密算法,如果使用不当,仍然可能被攻击者破解。因此,在实际应用中,我们需要使用更加复杂和安全的加密算法。
阅读全文
相关推荐
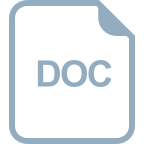
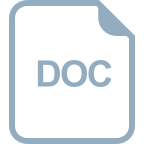
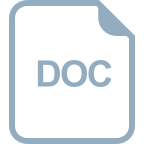

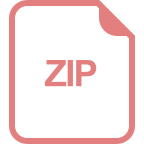
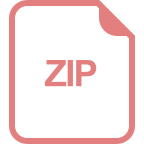

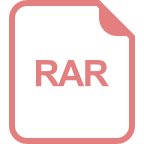


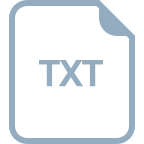
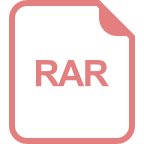
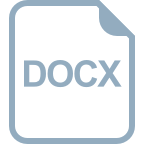
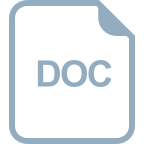

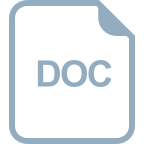
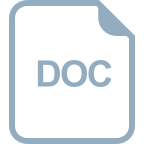
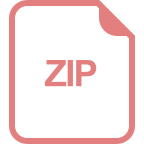
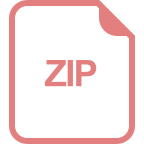