c语言实现仿射加密算法
时间: 2023-11-25 09:08:19 浏览: 48
仿射密码是一种基于数学原理的加密算法,它可以通过随机生成密钥a、b来加密和解密任意满足条件的数据。下面是一个基于C语言实现的仿射加密算法的示例代码:
```c
#include <stdio.h>
// 求两数的最大公约数
int gcd(int a, int b) {
return b ? gcd(b, a % b) : a;
}
// 求a模N=26的逆
int exgcd(int a, int n) {
int p = a, q = n;
int x = 0, y = 1;
int z = q / p;
while (p != 1 && q != 1) {
int t = p;
p = q % p;
q = t;
t = y;
y = x - y * z;
x = t;
z = q / p;
}
y %= n;
if (y < 0) y += n;
return y;
}
// 加密函数
void encrypt(char *plaintext, char *ciphertext, int a, int b) {
int i;
for (i = 0; plaintext[i] != '\0'; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = (a * (plaintext[i] - 'a') + b) % 26 + 'a';
} else if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
ciphertext[i] = (a * (plaintext[i] - 'A') + b) % 26 + 'A';
} else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[i] = '\0';
}
// 解密函数
void decrypt(char *ciphertext, char *plaintext, int a, int b) {
int i, a_inv = exgcd(a, 26);
for (i = 0; ciphertext[i] != '\0'; i++) {
if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z') {
plaintext[i] = (a_inv * (ciphertext[i] - 'a' - b + 26)) % 26 + 'a';
} else if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z') {
plaintext[i] = (a_inv * (ciphertext[i] - 'A' - b + 26)) % 26 + 'A';
} else {
plaintext[i] = ciphertext[i];
}
}
plaintext[i] = '\0';
}
int main() {
char plaintext[100], ciphertext[100], decrypted[100];
int a, b;
printf("请输入密钥a和b(用逗号隔开):");
scanf("%d,%d", &a, &b);
if (gcd(a, 26) != 1) {
printf("密钥a必须与26互质!\n");
return 0;
}
printf("请输入明文:");
scanf("%s", plaintext);
encrypt(plaintext, ciphertext, a, b);
printf("加密后的密文为:%s\n", ciphertext);
decrypt(ciphertext, decrypted, a, b);
printf("解密后的明文为:%s\n", decrypted);
return 0;
}
```
在这个示例代码中,我们定义了两个函数encrypt和decrypt,分别用于加密和解密数据。在加密时,我们将明文中的每个字符都转换为一个数字,然后使用公式(a * x + b) % 26来计算密文中的每个字符。在解密时,我们使用公式(a' * (y - b) % 26)来计算明文中的每个字符,其中a'是a模26的逆元。
相关推荐
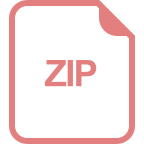
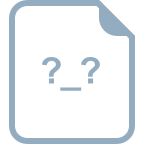












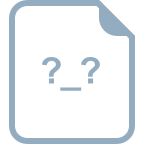