C语言 仿射密码加解密C语言实现代码。。。
时间: 2024-10-24 09:07:47 浏览: 28
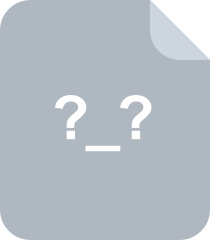
仿射密码 加解密 C语言

在C语言中,仿射密码是一种简单的替换加密算法,它通过将字母表中的每个字符按照一个固定的转换规则进行位移来加密和解密信息。下面是一个基本的仿射密码加解密的C语言实现示例:
```c
#include <stdio.h>
#include <string.h>
#define ALPHABET_SIZE 26
// 加密函数
char encrypt(char c, int key) {
if (c >= 'a' && c <= 'z') {
return ((c - 'a' + key) % ALPHABET_SIZE) + 'a';
} else if (c >= 'A' && c <= 'Z') {
return ((c - 'A' + key) % ALPHABET_SIZE) + 'A';
}
// 对非字母字符不做处理,保持原样
return c;
}
// 解密函数
char decrypt(char c, int key) {
return encrypt(c, -key);
}
int main() {
char plaintext[100], ciphertext[100];
int key;
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strlen(plaintext) - 1] = '\0'; // 去除换行符
printf("请输入加密钥:\n");
scanf("%d", &key);
for (int i = 0; plaintext[i]; ++i) {
ciphertext[i] = encrypt(plaintext[i], key);
}
ciphertext[strlen(ciphertext)] = '\0';
printf("加密后的密文是: %s\n", ciphertext);
printf("请输入解密密钥:\n");
scanf("%d", &key); // 使用相同的键解密
for (int i = 0; ciphertext[i]; ++i) {
plaintext[i] = decrypt(ciphertext[i], key);
}
plaintext[strlen(plaintext)] = '\0';
printf("解密后的原文是: %s\n", plaintext);
return 0;
}
```
在这个例子中,用户会被提示输入明文、加密键以及解密密钥。程序会分别对明文进行加密并输出密文,然后使用相同的解密密钥对密文进行解密。
阅读全文
相关推荐
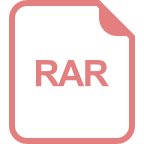
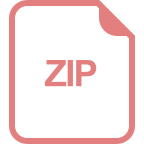

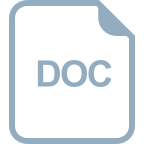


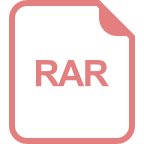
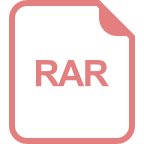
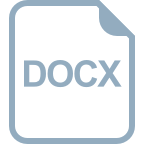
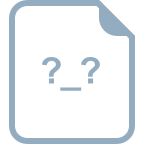






