c语言仿射变换加密解密代码
时间: 2023-09-09 13:00:34 浏览: 141
C语言中,可以通过仿射变换对数据进行加密和解密。仿射变换是一种线性变换,可以用于对字符或数据进行简单的置换和替换操作。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 加密函数
char* encrypt(char* message, int a, int b) {
int len = strlen(message);
char* encrypted = (char*)malloc(len * sizeof(char));
for (int i = 0; i < len; i++) {
if (message[i] >= 'a' && message[i] <= 'z') {
encrypted[i] = (char)((((a * (message[i] - 'a') + b) % 26) + 26) % 26 + 'a');
} else if (message[i] >= 'A' && message[i] <= 'Z') {
encrypted[i] = (char)((((a * (message[i] - 'A') + b) % 26) + 26) % 26 + 'A');
} else {
encrypted[i] = message[i];
}
}
return encrypted;
}
// 解密函数
char* decrypt(char* encrypted, int a, int b) {
int len = strlen(encrypted);
char* decrypted = (char*)malloc(len * sizeof(char));
int aInverse = 0;
// 计算a的模逆
for (int i = 0; i < 26; i++) {
if ((a * i) % 26 == 1) {
aInverse = i;
break;
}
}
for (int i = 0; i < len; i++) {
if (encrypted[i] >= 'a' && encrypted[i] <= 'z') {
decrypted[i] = (char)((((aInverse * ((encrypted[i] - 'a') - b + 26)) % 26) + 26) % 26 + 'a');
} else if (encrypted[i] >= 'A' && encrypted[i] <= 'Z') {
decrypted[i] = (char)((((aInverse * ((encrypted[i] - 'A') - b + 26)) % 26) + 26) % 26 + 'A');
} else {
decrypted[i] = encrypted[i];
}
}
return decrypted;
}
int main() {
char message[100];
int a, b;
printf("请输入要加密的消息:");
scanf("%[^\n]", message);
getchar(); // 清除输入缓冲区的换行符
printf("请输入加密参数a和b(以空格分隔):");
scanf("%d %d", &a, &b);
char* encrypted = encrypt(message, a, b);
printf("加密结果:%s\n", encrypted);
char* decrypted = decrypt(encrypted, a, b);
printf("解密结果:%s\n", decrypted);
free(encrypted);
free(decrypted);
return 0;
}
```
以上代码实现了仿射变换的加密和解密功能。用户可以输入要加密的消息和加密参数a、b,程序将对消息进行加密并输出加密结果,然后再对加密结果进行解密并输出解密结果。加密过程中,对于字母字符,将其根据仿射变换的置换和替换公式进行操作;对于非字母字符,直接保留不变。解密过程中,先通过计算a的模逆找到解密用的参数,再对加密结果进行反操作,恢复原始消息。
阅读全文
相关推荐








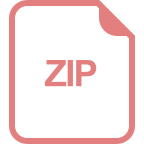
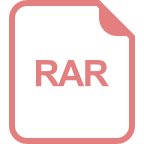







