用C语言编写一个实现仿射密码加密和解密功能的程序
时间: 2024-12-18 16:33:29 浏览: 9
在C语言中,我们可以创建一个简单的仿射密码(Affine Cipher)加密和解密程序,仿射密码是一种替换式加密,基于两个公共整数(a和b)。加密过程将每个字母转换为其字母表中的对应位置乘以a再加上b的结果,再对字母表长度取模,以防止超出字母范围。下面是这个程序的基本结构:
```c
#include <stdio.h>
#include <ctype.h> // 包含isalpha函数
// 定义字母表大小
#define ALPHABET_SIZE 26
// 加密函数
char affine_encrypt(char c, int a, int b) {
if (isalpha(c)) { // 只对字母进行操作
int index = tolower(c) - 'a'; // 将字母转换为小写字母并计算其在字母表的位置
return (index * a + b) % ALPHABET_SIZE + 'a';
}
return c; // 非字母字符不做处理,直接返回
}
// 解密函数
char affine_decrypt(char c, int a, int b) {
return affine_encrypt(c, a, -b); // 解密时只需改变加法符号
}
int main() {
char plaintext[100], ciphertext[100];
int a, b;
printf("请输入加密系数a和b (1-25): ");
scanf("%d %d", &a, &b);
printf("Enter the plain text: ");
fgets(plaintext, sizeof(plaintext), stdin); // 防止换行符
plaintext[strlen(plaintext)-1] = '\0'; // 去除fgets产生的换行符
for (int i = 0; plaintext[i]; ++i) {
ciphertext[i] = affine_encrypt(plaintext[i], a, b);
}
ciphertext[strlen(ciphertext)] = '\0'; // 添加终止符
printf("Encrypted message: %s\n", ciphertext);
printf("Enter the encrypted text: ");
fgets(ciphertext, sizeof(ciphertext), stdin); // 读入加密文本
ciphertext[strlen(ciphertext)-1] = '\0';
for (int i = 0; ciphertext[i]; ++i) {
plaintext[i] = affine_decrypt(ciphertext[i], a, b);
}
plaintext[strlen(plaintext)] = '\0';
printf("Decrypted message: %s\n", plaintext);
return 0;
}
```
在这个程序中,用户需要提供加密系数a和b,然后输入明文进行加密,再输入加密后的密文进行解密。注意,这个程序仅对英文字符有效,并假设只有字母参与加密和解密过程。
阅读全文
相关推荐
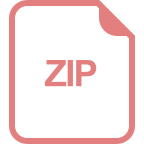
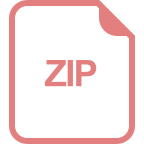

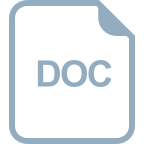








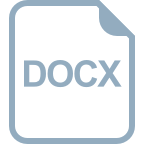
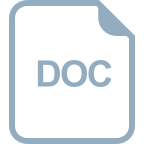



