在C++当中用代码实现仿射密码的加密和解密
时间: 2023-05-31 19:01:43 浏览: 119
下面是C语言实现仿射密码的加密和解密的代码:
```
#include <stdio.h>
#include <string.h>
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
int modInverse(int a, int m) {
a = a % m;
for (int x = 1; x < m; x++) {
if ((a * x) % m == 1) {
return x;
}
}
return -1;
}
void affine_encrypt(char* plaintext, int a, int b, char* ciphertext) {
int len = strlen(plaintext);
for (int i = 0; i < len; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = (char) (((a * (plaintext[i] - 'a') + b) % 26) + 'a');
} else if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
ciphertext[i] = (char) (((a * (plaintext[i] - 'A') + b) % 26) + 'A');
} else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[len] = '\0';
}
void affine_decrypt(char* ciphertext, int a, int b, char* plaintext) {
int len = strlen(ciphertext);
int a_inv = modInverse(a, 26);
for (int i = 0; i < len; i++) {
if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z') {
plaintext[i] = (char) (((a_inv * ((ciphertext[i] - 'a' - b + 26) % 26)) % 26) + 'a');
} else if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z') {
plaintext[i] = (char) (((a_inv * ((ciphertext[i] - 'A' - b + 26) % 26)) % 26) + 'A');
} else {
plaintext[i] = ciphertext[i];
}
}
plaintext[len] = '\0';
}
int main() {
char plaintext[100], ciphertext[100], decryptedtext[100];
int a, b;
printf("Enter the plaintext: ");
scanf("%[^\n]s", plaintext);
printf("Enter the value of a (should be coprime with 26): ");
scanf("%d", &a);
printf("Enter the value of b: ");
scanf("%d", &b);
if (gcd(a, 26) != 1) {
printf("Invalid value of a. It should be coprime with 26.\n");
return 0;
}
affine_encrypt(plaintext, a, b, ciphertext);
printf("Encrypted text: %s\n", ciphertext);
affine_decrypt(ciphertext, a, b, decryptedtext);
printf("Decrypted text: %s\n", decryptedtext);
return 0;
}
```
在这个程序中,我们使用了两个函数affine_encrypt和affine_decrypt来分别实现仿射密码的加密和解密。这两个函数的参数分别是明文,a和b(这是仿射密码的两个参数),以及密文或解密后的明文。我们还使用了gcd函数来计算两个数的最大公约数,以及modInverse函数来计算a模26的逆元。在主函数中,我们先输入明文,a和b,然后进行加密和解密,并输出结果。注意,输入的明文应该只包含字母和空格,不应该包含标点符号或数字。
阅读全文
相关推荐



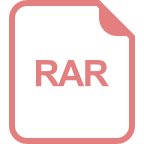










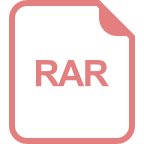