如何在Python中实现维吉尼亚加密算法,并确保加密后的密文能正确解密回原始明文?
时间: 2024-11-15 22:18:22 浏览: 24
要实现维吉尼亚加密算法,首先需要了解算法的基本工作原理。维吉尼亚算法是一种多表代换密码,它通过一个重复使用的密钥来加密明文,每个密钥字符对应一个字母表的位移。实现这一算法的关键在于正确处理明文和密钥的字符编码,以及实现加密和解密的数学运算。
参考资源链接:[Python编程实现维吉尼亚加密算法详解](https://wenku.csdn.net/doc/7k363eyjaz?spm=1055.2569.3001.10343)
在Python中,我们可以创建一个函数来处理加密和解密过程。首先,需要确保输入的明文和密钥都是大写字母,然后建立一个字符编码表,通常使用英文字母表A到Z,每个字母对应一个数字(A=0, B=1, ..., Z=25)。接着,我们通过遍历明文和密钥,应用维吉尼亚算法的加密公式进行加密,加密公式为 \( C_j = (M_j + K_j) \mod 26 \),其中 \( C_j \) 是第j个密文字符,\( M_j \) 是第j个明文字符,\( K_j \) 是第j个密钥字符。
解密过程则需要调整公式,使得 \( M_j = (C_j - K_j + 26) \mod 26 \),这样可以得到正确的明文字符。由于Python中的整数除法会舍去小数部分,因此在解密时加上26是为了确保负数索引能够正确映射回字母表中。
在实现时,我们还需要处理密钥长度与明文长度不一致的情况。一种常见的方法是让密钥重复直到与明文长度相匹配。对于非字母字符,可以保留原样或选择性地去除。
以下是一个简化的Python代码示例,展示了如何实现维吉尼亚加密和解密:
```python
def vigenere_encrypt(plaintext, key):
key = (key * (len(plaintext) // len(key) + 1))[:len(plaintext)]
ciphertext = ''
for i in range(len(plaintext)):
if plaintext[i].isalpha():
offset = 65 if plaintext[i].isupper() else 97
char_int = ord(plaintext[i]) - offset
key_int = ord(key[i]) - offset
ciphertext += chr((char_int + key_int) % 26 + offset)
else:
ciphertext += plaintext[i]
return ciphertext
def vigenere_decrypt(ciphertext, key):
key = (key * (len(ciphertext) // len(key) + 1))[:len(ciphertext)]
plaintext = ''
for i in range(len(ciphertext)):
if ciphertext[i].isalpha():
offset = 65 if ciphertext[i].isupper() else 97
char_int = ord(ciphertext[i]) - offset
key_int = ord(key[i]) - offset
plaintext += chr((char_int - key_int + 26) % 26 + offset)
else:
plaintext += ciphertext[i]
return plaintext
# 示例使用
key =
参考资源链接:[Python编程实现维吉尼亚加密算法详解](https://wenku.csdn.net/doc/7k363eyjaz?spm=1055.2569.3001.10343)
阅读全文
相关推荐
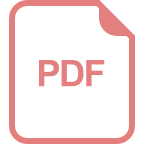
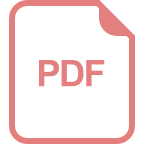
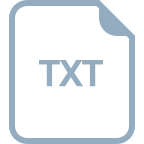

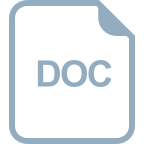
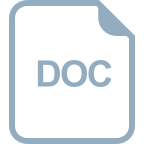
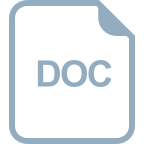
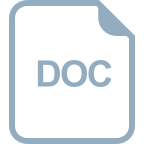








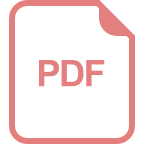
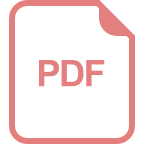