c语言实现Vigenere密码算法
时间: 2023-10-04 07:14:07 浏览: 125
以下是C语言实现Vigenere密码算法的示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
char* encrypt(char* plaintext, char* key);
char* decrypt(char* ciphertext, char* key);
int main() {
char plaintext[MAX_LEN];
char key[MAX_LEN];
char ciphertext[MAX_LEN];
printf("Enter plaintext: ");
fgets(plaintext, MAX_LEN, stdin);
plaintext[strcspn(plaintext, "\n")] = 0; // remove newline character
printf("Enter key: ");
fgets(key, MAX_LEN, stdin);
key[strcspn(key, "\n")] = 0; // remove newline character
strcpy(ciphertext, encrypt(plaintext, key));
printf("Ciphertext: %s\n", ciphertext);
strcpy(plaintext, decrypt(ciphertext, key));
printf("Decrypted plaintext: %s\n", plaintext);
return 0;
}
char* encrypt(char* plaintext, char* key) {
int plaintext_len = strlen(plaintext);
int key_len = strlen(key);
char* ciphertext = malloc(plaintext_len + 1);
for (int i = 0; i < plaintext_len; i++) {
ciphertext[i] = ((plaintext[i] - 'A') + (key[i % key_len] - 'A')) % 26 + 'A';
}
ciphertext[plaintext_len] = '\0';
return ciphertext;
}
char* decrypt(char* ciphertext, char* key) {
int ciphertext_len = strlen(ciphertext);
int key_len = strlen(key);
char* plaintext = malloc(ciphertext_len + 1);
for (int i = 0; i < ciphertext_len; i++) {
plaintext[i] = ((ciphertext[i] - 'A') - (key[i % key_len] - 'A') + 26) % 26 + 'A';
}
plaintext[ciphertext_len] = '\0';
return plaintext;
}
```
该程序从用户输入获取明文和密钥,并使用`encrypt()`函数加密明文并返回密文,使用`decrypt()`函数解密密文并返回明文。在加密和解密过程中,程序将明文和密文中的字母从'A'到'Z'映射到0到25,执行Vigenere算法,然后将结果映射回字母形式。请注意,该程序假定输入的明文和密钥都是大写字母。
相关推荐
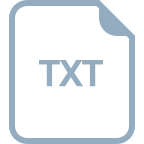














