c语言vigenere解密文件
时间: 2023-03-21 10:03:29 浏览: 90
可以的,关于C语言的Vigenere解密文件,您可以参考以下步骤:
1. 首先,您需要编写一个C语言程序,该程序需要读取需要解密的文件。
2. 然后,您需要定义一个密钥,该密钥将用于Vigenere解密。
3. 接下来,您需要使用Vigenere解密算法对文件进行解密。您可以使用以下步骤:
a. 读取文件中的每个字符,并将其转换为对应的ASCII码值。
b. 将密钥中的每个字符也转换为对应的ASCII码值。
c. 对于文件中的每个字符,使用Vigenere解密算法进行解密。具体来说,您可以使用以下公式:
plaintext = (ciphertext - key + 26) % 26 + 'A';
其中,ciphertext是密文字符的ASCII码值,key是密钥字符的ASCII码值,plaintext是明文字符的ASCII码值。
d. 将解密后的明文字符写入一个新的文件中。
4. 最后,您需要关闭所有文件并释放内存。
希望这些步骤能够帮助您解决C语言的Vigenere解密文件问题。
相关问题
用C语言编写 Vigenere 密码解密程序,在已知密钥的情况下将密文文件解密得到明文文件
以下是用C语言实现Vigenere密码解密程序的示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 1000 // 最大文件长度
int main() {
char key[] = "SECRETKEY"; // 密钥
char cipher_text[MAX_LEN]; // 密文
char plain_text[MAX_LEN]; // 明文
char ch;
int key_len = strlen(key);
int i, j;
// 读取密文文件
FILE *fp_cipher = fopen("cipher.txt", "r");
if (fp_cipher == NULL) {
printf("Error opening cipher file.\n");
return 1;
}
i = 0;
while ((ch = fgetc(fp_cipher)) != EOF) {
cipher_text[i++] = ch;
}
cipher_text[i] = '\0';
fclose(fp_cipher);
// 解密
for (i = 0, j = 0; i < strlen(cipher_text); i++, j++) {
if (j == key_len) j = 0;
int shift = key[j] - 'A';
if (cipher_text[i] >= 'A' && cipher_text[i] <= 'Z') {
plain_text[i] = (cipher_text[i] - shift - 'A' + 26) % 26 + 'A';
} else if (cipher_text[i] >= 'a' && cipher_text[i] <= 'z') {
plain_text[i] = (cipher_text[i] - shift - 'a' + 26) % 26 + 'a';
} else {
plain_text[i] = cipher_text[i];
j--;
}
}
plain_text[i] = '\0';
// 写入明文文件
FILE *fp_plain = fopen("plain.txt", "w");
if (fp_plain == NULL) {
printf("Error opening plain file.\n");
return 1;
}
fprintf(fp_plain, "%s", plain_text);
fclose(fp_plain);
printf("Decryption finished.\n");
return 0;
}
```
在这个程序中,我们首先读取密文文件“cipher.txt”,然后使用给定的密钥解密密文,得到明文。解密过程中,我们使用循环密钥方法,即每次使用密钥中的下一个字符进行加密,直到整个密文都被解密。在解密过程中,我们需要注意处理非字母字符(如空格、标点符号等),这些字符不需要进行解密操作。最后,我们将得到的明文写入“plain.txt”文件中。
需要注意的是,这个程序并没有进行输入合法性检查,例如密文文件不存在、密钥为空等情况都没有进行异常处理。在实际应用中,我们需要进行充分的输入合法性检查和异常处理,以确保程序的正确性和稳定性。
c语言实现Vigenere密码算法
以下是C语言实现Vigenere密码算法的示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
char* encrypt(char* plaintext, char* key);
char* decrypt(char* ciphertext, char* key);
int main() {
char plaintext[MAX_LEN];
char key[MAX_LEN];
char ciphertext[MAX_LEN];
printf("Enter plaintext: ");
fgets(plaintext, MAX_LEN, stdin);
plaintext[strcspn(plaintext, "\n")] = 0; // remove newline character
printf("Enter key: ");
fgets(key, MAX_LEN, stdin);
key[strcspn(key, "\n")] = 0; // remove newline character
strcpy(ciphertext, encrypt(plaintext, key));
printf("Ciphertext: %s\n", ciphertext);
strcpy(plaintext, decrypt(ciphertext, key));
printf("Decrypted plaintext: %s\n", plaintext);
return 0;
}
char* encrypt(char* plaintext, char* key) {
int plaintext_len = strlen(plaintext);
int key_len = strlen(key);
char* ciphertext = malloc(plaintext_len + 1);
for (int i = 0; i < plaintext_len; i++) {
ciphertext[i] = ((plaintext[i] - 'A') + (key[i % key_len] - 'A')) % 26 + 'A';
}
ciphertext[plaintext_len] = '\0';
return ciphertext;
}
char* decrypt(char* ciphertext, char* key) {
int ciphertext_len = strlen(ciphertext);
int key_len = strlen(key);
char* plaintext = malloc(ciphertext_len + 1);
for (int i = 0; i < ciphertext_len; i++) {
plaintext[i] = ((ciphertext[i] - 'A') - (key[i % key_len] - 'A') + 26) % 26 + 'A';
}
plaintext[ciphertext_len] = '\0';
return plaintext;
}
```
该程序从用户输入获取明文和密钥,并使用`encrypt()`函数加密明文并返回密文,使用`decrypt()`函数解密密文并返回明文。在加密和解密过程中,程序将明文和密文中的字母从'A'到'Z'映射到0到25,执行Vigenere算法,然后将结果映射回字母形式。请注意,该程序假定输入的明文和密钥都是大写字母。
相关推荐
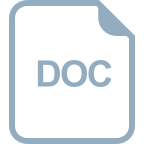








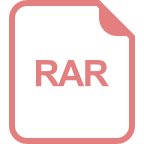
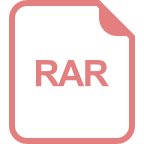