用C语言编写 Vigenere 密码解密程序,在已知密钥的情况下将密文文件解密得到明文文件
时间: 2023-06-01 14:03:12 浏览: 66
以下是用C语言实现Vigenere密码解密程序的示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 1000 // 最大文件长度
int main() {
char key[] = "SECRETKEY"; // 密钥
char cipher_text[MAX_LEN]; // 密文
char plain_text[MAX_LEN]; // 明文
char ch;
int key_len = strlen(key);
int i, j;
// 读取密文文件
FILE *fp_cipher = fopen("cipher.txt", "r");
if (fp_cipher == NULL) {
printf("Error opening cipher file.\n");
return 1;
}
i = 0;
while ((ch = fgetc(fp_cipher)) != EOF) {
cipher_text[i++] = ch;
}
cipher_text[i] = '\0';
fclose(fp_cipher);
// 解密
for (i = 0, j = 0; i < strlen(cipher_text); i++, j++) {
if (j == key_len) j = 0;
int shift = key[j] - 'A';
if (cipher_text[i] >= 'A' && cipher_text[i] <= 'Z') {
plain_text[i] = (cipher_text[i] - shift - 'A' + 26) % 26 + 'A';
} else if (cipher_text[i] >= 'a' && cipher_text[i] <= 'z') {
plain_text[i] = (cipher_text[i] - shift - 'a' + 26) % 26 + 'a';
} else {
plain_text[i] = cipher_text[i];
j--;
}
}
plain_text[i] = '\0';
// 写入明文文件
FILE *fp_plain = fopen("plain.txt", "w");
if (fp_plain == NULL) {
printf("Error opening plain file.\n");
return 1;
}
fprintf(fp_plain, "%s", plain_text);
fclose(fp_plain);
printf("Decryption finished.\n");
return 0;
}
```
在这个程序中,我们首先读取密文文件“cipher.txt”,然后使用给定的密钥解密密文,得到明文。解密过程中,我们使用循环密钥方法,即每次使用密钥中的下一个字符进行加密,直到整个密文都被解密。在解密过程中,我们需要注意处理非字母字符(如空格、标点符号等),这些字符不需要进行解密操作。最后,我们将得到的明文写入“plain.txt”文件中。
需要注意的是,这个程序并没有进行输入合法性检查,例如密文文件不存在、密钥为空等情况都没有进行异常处理。在实际应用中,我们需要进行充分的输入合法性检查和异常处理,以确保程序的正确性和稳定性。
相关推荐
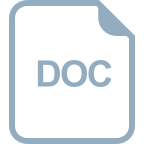














