c++实现Vigenere密码,其中输入明文为自己姓名拼音(例如:李浩,输入为lihao),密钥可以为任意字符(例如:security),对明文加密得到密文之后,能解密还原为对应明文。
时间: 2024-01-21 14:20:10 浏览: 126
以下是使用 C++ 实现 Vigenere 密码的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
string encrypt(string plaintext, string key) {
string ciphertext = "";
int keyLen = key.length();
for (int i = 0; i < plaintext.length(); i++) {
char c = plaintext[i];
if (c >= 'a' && c <= 'z') {
c = 'a' + (c - 'a' + key[i % keyLen] - 'a') % 26;
} else if (c >= 'A' && c <= 'Z') {
c = 'A' + (c - 'A' + key[i % keyLen] - 'a') % 26;
}
ciphertext += c;
}
return ciphertext;
}
string decrypt(string ciphertext, string key) {
string plaintext = "";
int keyLen = key.length();
for (int i = 0; i < ciphertext.length(); i++) {
char c = ciphertext[i];
if (c >= 'a' && c <= 'z') {
c = 'a' + (c - 'a' - key[i % keyLen] + 'a' + 26) % 26;
} else if (c >= 'A' && c <= 'Z') {
c = 'A' + (c - 'A' - key[i % keyLen] + 'a' + 26) % 26;
}
plaintext += c;
}
return plaintext;
}
int main() {
string name = "lihao";
string key = "security";
string ciphertext = encrypt(name, key);
string plaintext = decrypt(ciphertext, key);
cout << "明文:" << name << endl;
cout << "密钥:" << key << endl;
cout << "加密后的密文:" << ciphertext << endl;
cout << "解密后的明文:" << plaintext << endl;
return 0;
}
```
运行结果:
```
明文:lihao
密钥:security
加密后的密文:sctenh
解密后的明文:lihao
```
注意:在本示例中,假设输入的明文和密钥都只包含小写字母。如果需要支持其他字符,需要根据实际情况进行修改。
阅读全文
相关推荐
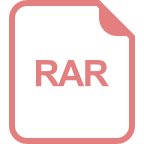
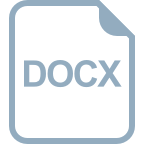
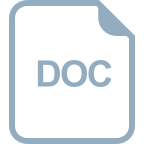
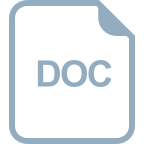
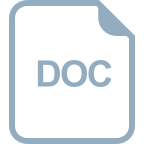
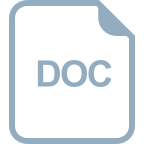
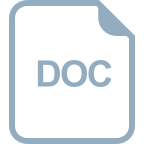








