写一个维吉尼亚密码——加密的python程序,要求输入为两行,第一行是密钥secret_key,第二行是明文plain_text。
时间: 2023-11-27 16:51:40 浏览: 59
以下是维吉尼亚密码的加密Python程序:
```python
def encrypt_vigenere(secret_key, plain_text):
"""
使用维吉尼亚密码加密明文。
参数:
secret_key -- 密钥,为一个字符串。
plain_text -- 明文,为一个字符串。
返回值:
密文,为一个字符串。
"""
# 将密钥和明文转化为大写字母
secret_key = secret_key.upper()
plain_text = plain_text.upper()
# 生成密钥序列
key_stream = ''
key_index = 0
for i in range(len(plain_text)):
# 如果字符不是字母,则忽略
if not plain_text[i].isalpha():
key_stream += plain_text[i]
else:
key_stream += secret_key[key_index]
key_index = (key_index + 1) % len(secret_key)
# 加密明文
cipher_text = ''
for i in range(len(plain_text)):
# 如果字符不是字母,则保留原样
if not plain_text[i].isalpha():
cipher_text += plain_text[i]
else:
# 计算加密后的字符
shift = ord(key_stream[i]) - ord('A')
cipher_char = chr((ord(plain_text[i]) - ord('A') + shift) % 26 + ord('A'))
cipher_text += cipher_char
return cipher_text
```
使用示例:
```python
secret_key = input() # 输入密钥
plain_text = input() # 输入明文
cipher_text = encrypt_vigenere(secret_key, plain_text)
print(cipher_text)
```
注意,该程序仅支持英文字母的加密,其他字符会被保留原样,并且会忽略密钥和明文中的空格和标点符号。如果需要支持更多字符,可以修改代码中的字符集。
阅读全文
相关推荐
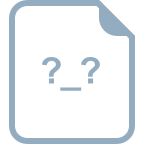













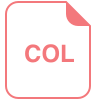

