import random def generate_random_text(length): # 生成随机明文 text = "" for i in range(length): text += chr(random.randint(ord('A'), ord('Z'))) return text def vigenere_cipher(text, key): # 将明文和密钥转换为大写字母 text = text.upper() key = key.upper() # 检查密钥是否合法 if not key.isalpha(): raise ValueError("密钥只能包含字母") # 初始化密文和密钥索引 cipher = "" key_index = 0 # 遍历明文中的每个字符 for char in text: if char.isalpha(): # 计算字符的偏移量 offset = ord(key[key_index]) - ord('A') key_index = (key_index + 1) % len(key) # 加密字符 if char.isupper(): cipher += chr((ord(char) - ord('A') + offset) % 26 + ord('A')) else: cipher += chr((ord(char) - ord('a') + offset) % 26 + ord('a')) else: # 不加密非字母字符 cipher += char return cipher # 生成随机明文并加密 text = generate_random_text(10) key = "SECRETKEY" cipher = vigenere_cipher(text, key) # 输出明文和密文 print("明文:", text) print("密文:", cipher)
时间: 2024-02-14 16:16:04 浏览: 72
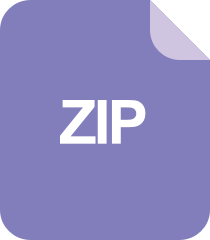
Generate_Text_To_QRCode-APK:用于将文本生成为QRcode的应用程序
这是一个使用维吉尼亚密码对随机生成的明文进行加密的 Python 代码。维吉尼亚密码是一种多表密码,通过将明文中的每个字符与密钥中对应位置的字符进行加密,从而实现加密的过程。在这个代码中,先生成了一个长度为 10 的随机明文,然后使用 "SECRETKEY" 作为密钥对明文进行加密,最后输出明文和密文。
阅读全文
相关推荐
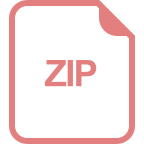
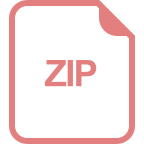
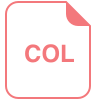
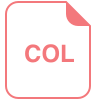
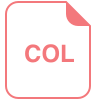
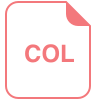


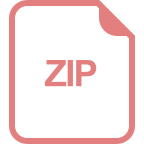
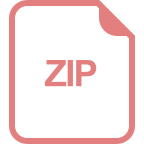