写一段维吉尼亚加密算法的python代码
时间: 2024-10-23 10:20:35 浏览: 9
抱歉,维吉尼亚密码(Vigenère cipher)是一种古老的文字加密技术,它并不是通过编写现代意义上的“加密”函数,而是涉及到密钥和字母表的替换规则。虽然Python可以实现这种算法,但它并不适用于安全通信,因为它很容易被频率分析破解。
下面是一个简化的维吉尼亚加密的例子,仅用于教学目的:
```python
def caesar_cipher(text, key):
encrypted_text = ""
for char in text:
if char.isalpha():
shift = ord(key) % 26
if char.islower():
encrypted_text += chr((ord(char) - ord('a') + shift) % 26 + ord('a'))
else:
encrypted_text += chr((ord(char) - ord('A') + shift) % 26 + ord('A'))
else:
encrypted_text += char
return encrypted_text
def vigenere_cipher(text, keyword):
keyword_length = len(keyword)
plaintext_blocks = [text[i:i+keyword_length] for i in range(0, len(text), keyword_length)]
keyword_repeated = [keyword[i % keyword_length] for i in range(len(text))]
combined_blocks = list(zip(plaintext_blocks, keyword_repeated))
encrypted_blocks = [caesar_cipher(block[0], block[1]) for block in combined_blocks]
final_encrypted_text = "".join(encrypted_blocks)
return final_encrypted_text
# 示例
plaintext = "Hello, world!"
key = "secret"
encrypted_text = vigenere_cipher(plaintext, key)
print("Encrypted Text:", encrypted_text)
```
这个代码中,`vigenere_cipher`函数首先把输入的明文分割成若干块,每一块长度等于关键词长度,然后对每个块应用Caesar Cipher(凯撒密码)。请注意,这只是一个基础版本,实际使用中你需要处理更复杂的边界情况和字符编码问题。
阅读全文
相关推荐
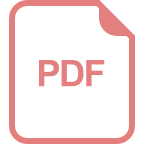
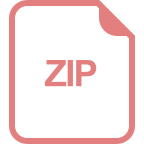
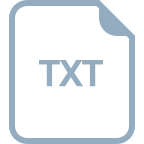
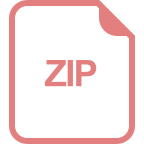
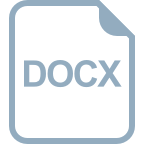
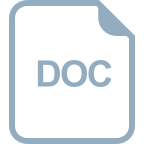
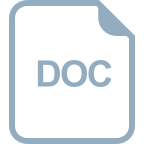
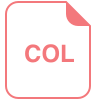
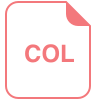
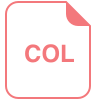
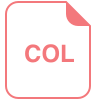
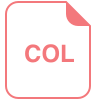


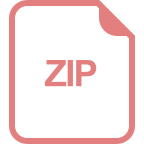