4. 用Vigenere密码算法加解密文本文件中的文本信息,设密钥长度为7,密钥在程序执行时动态输入,加密得到的密文放在另一个文本文件中,设计的程序要有界面
时间: 2024-02-12 11:02:26 浏览: 113
好的,这是一个比较复杂的问题,需要用到一些编程知识和算法。以下是一个简单的Python程序,可以实现Vigenere密码算法加解密文本文件中的文本信息,同时具有GUI界面。
```python
import tkinter as tk
from tkinter import filedialog
def encrypt(key, plaintext):
ciphertext = ""
key_len = len(key)
for i in range(len(plaintext)):
letter = plaintext[i]
shift = ord(key[i % key_len]) - ord('a')
if letter.isalpha():
if letter.isupper():
ciphertext += chr((ord(letter) - ord('A') + shift) % 26 + ord('A'))
else:
ciphertext += chr((ord(letter) - ord('a') + shift) % 26 + ord('a'))
else:
ciphertext += letter
return ciphertext
def decrypt(key, ciphertext):
plaintext = ""
key_len = len(key)
for i in range(len(ciphertext)):
letter = ciphertext[i]
shift = ord(key[i % key_len]) - ord('a')
if letter.isalpha():
if letter.isupper():
plaintext += chr((ord(letter) - ord('A') - shift + 26) % 26 + ord('A'))
else:
plaintext += chr((ord(letter) - ord('a') - shift + 26) % 26 + ord('a'))
else:
plaintext += letter
return plaintext
def select_file(textvar):
file_path = filedialog.askopenfilename()
textvar.set(file_path)
def run():
key = key_entry.get()
input_file = input_file_var.get()
output_file = output_file_var.get()
with open(input_file, 'r') as f:
plaintext = f.read()
ciphertext = encrypt(key, plaintext)
with open(output_file, 'w') as f:
f.write(ciphertext)
status_label.config(text="Done.")
def run_decrypt():
key = key_entry.get()
input_file = input_file_var.get()
output_file = output_file_var.get()
with open(input_file, 'r') as f:
ciphertext = f.read()
plaintext = decrypt(key, ciphertext)
with open(output_file, 'w') as f:
f.write(plaintext)
status_label.config(text="Done.")
root = tk.Tk()
key_label = tk.Label(root, text="Key:")
key_label.grid(row=0, column=0)
key_entry = tk.Entry(root)
key_entry.grid(row=0, column=1)
input_file_label = tk.Label(root, text="Input File:")
input_file_label.grid(row=1, column=0)
input_file_var = tk.StringVar()
input_file_entry = tk.Entry(root, textvariable=input_file_var)
input_file_entry.grid(row=1, column=1)
input_file_button = tk.Button(root, text="Select File", command=lambda: select_file(input_file_var))
input_file_button.grid(row=1, column=2)
output_file_label = tk.Label(root, text="Output File:")
output_file_label.grid(row=2, column=0)
output_file_var = tk.StringVar()
output_file_entry = tk.Entry(root, textvariable=output_file_var)
output_file_entry.grid(row=2, column=1)
output_file_button = tk.Button(root, text="Select File", command=lambda: select_file(output_file_var))
output_file_button.grid(row=2, column=2)
encrypt_button = tk.Button(root, text="Encrypt", command=run)
encrypt_button.grid(row=3, column=0)
decrypt_button = tk.Button(root, text="Decrypt", command=run_decrypt)
decrypt_button.grid(row=3, column=1)
status_label = tk.Label(root, text="")
status_label.grid(row=4, column=0, columnspan=3)
root.mainloop()
```
这个程序使用了Tkinter模块来创建GUI界面,包括输入密钥、选择输入文件和输出文件等功能。当用户点击“Encrypt”按钮时,程序会读取输入文件中的文本信息,使用Vigenere密码算法加密,并将密文写入输出文件中。当用户点击“Decrypt”按钮时,程序会读取输入文件中的密文,使用Vigenere密码算法解密,并将明文写入输出文件中。注意,需要先输入密钥才能进行加解密操作。
希望这个程序能够帮助到你,如果有任何问题,请随时联系我。
阅读全文
相关推荐



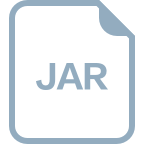











