用java完成给定整数数组arr = [2,3,5,3,2,7,6,6,6,3,3,3,7,5,4,2,9,6,4,2,8,6,9,1,3,6,8,1,1,5,3,6,6,6,7,7,8]。从数组中找出出现频率最高的前 4个数字,并返回这四个数字以及对应的频数
时间: 2024-09-15 09:13:11 浏览: 30
在Java中,我们可以使用HashMap来存储每个数字及其出现的次数,然后遍历一次数组就可以找到出现频率最高的前四个数字。以下是简单的步骤:
1. 创建一个HashMap来存储数字和它们的出现次数。
2. 遍历输入数组`arr`,对于每个元素,如果它已经在HashMap中,则增加其计数;如果不在,则添加新的键值对(数字作为键,初始计数为1)。
3. 使用PriorityQueue(堆数据结构),将HashMap中的键值对按照值(即出现次数)降序排列,同时限制容量为4。
4. 从PriorityQueue中获取并删除最大的元素四次,直到队列为空,此时队列中的元素就是出现频率最高的前四个数字及其对应的频数。
以下是一个示例Java代码来实现这个功能:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
int[] arr = {2, 3, 5, 3, 2, 7, 6, 6, 6, 3, 3, 3, 7, 5, 4, 2, 9, 6, 4, 2, 8, 6, 9, 1, 3, 6, 8, 1, 1, 5, 3, 6, 6, 6, 7, 7, 8};
Map<Integer, Integer> freqMap = new HashMap<>();
for (int num : arr) {
freqMap.put(num, freqMap.getOrDefault(num, 0) + 1);
}
PriorityQueue<Map.Entry<Integer, Integer>> pq = new PriorityQueue<>((a, b) -> b.getValue() - a.getValue());
for (Map.Entry<Integer, Integer> entry : freqMap.entrySet()) {
pq.offer(entry);
if (pq.size() > 4) {
pq.poll();
}
}
System.out.println("出现频率最高的前4个数字及对应频数:");
while (!pq.isEmpty()) {
Map.Entry<Integer, Integer> topEntry = pq.poll();
System.out.format("%d 出现了 %d 次%n", topEntry.getKey(), topEntry.getValue());
}
}
}
```
相关推荐
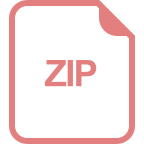
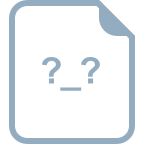
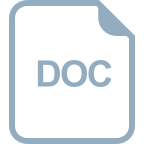














