Python 解析位域结构体
时间: 2023-12-05 20:39:31 浏览: 206
位域结构体是一种特殊的结构体,它的成员变量可以指定占用的位数,从而实现对内存的更加精细的控制。在Python中,可以使用ctypes库来解析位域结构体。具体步骤如下:
1. 导入ctypes库
```python
import ctypes
```
2. 定义位域结构体
```python
class CANFrame(ctypes.LittleEndianStructure):
_fields_ = [
("can_id", ctypes.c_uint32, 11),
("can_dlc", ctypes.c_uint32, 4),
("pad", ctypes.c_uint32, 1),
("res0", ctypes.c_uint32, 1),
("res1", ctypes.c_uint32, 1),
("can_rtr", ctypes.c_uint32, 1),
("can_err", ctypes.c_uint32, 1),
("can_ext", ctypes.c_uint32, 1),
("__pad", ctypes.c_uint32, 1),
("__res0", ctypes.c_uint32, 1),
("__res1", ctypes.c_uint32, 1),
("__res2", ctypes.c_uint32, 1),
("__res3", ctypes.c_uint32, 1),
("__res4", ctypes.c_uint32, 1),
("__res5", ctypes.c_uint32, 1),
("__res6", ctypes.c_uint32, 1),
("data", ctypes.c_uint8 * 8)
]
```
3. 解析CAN帧数据
```python
# 假设收到的CAN帧数据为b'\x00\x00\x00\x00\x08\x00\x00\x00\x01\x02\x03\x04\x05\x06\x07\x08'
can_frame = CANFrame.from_buffer_copy(b'\x00\x00\x00\x00\x08\x00\x00\x00\x01\x02\x03\x04\x05\x06\x07\x08')
# 访问CAN帧数据的成员变量
print(can_frame.can_id) # 输出:0
print(can_frame.can_dlc) # 输出:8
print(can_frame.data) # 输出:[1, 2, 3, 4, 5, 6, 7, 8]
```
在上述代码中,我们首先定义了一个名为CANFrame的位域结构体,其中包含了CAN帧的各个字段。然后,我们使用from_buffer_copy方法将收到的CAN帧数据转换为CANFrame类型的对象,从而可以方便地访问其中的各个成员变量。
阅读全文
相关推荐
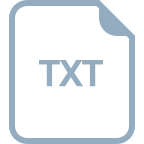


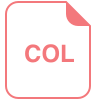
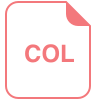
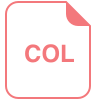
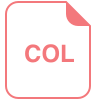
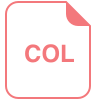
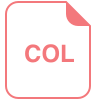
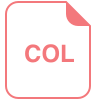
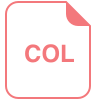
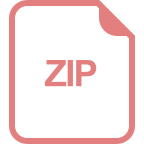
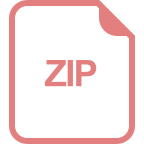
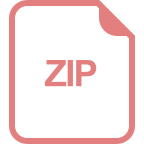