实现Promise.retry:执行一个函数,如果不成功最多可以尝试 times 次。传参需要三个变量,所要执行的函数,尝试的次数以及延迟的时间。
时间: 2024-04-12 22:28:56 浏览: 170
你可以通过以下方式实现`Promise.retry`函数:
```javascript
function retry(fn, times, delay) {
return new Promise((resolve, reject) => {
const attempt = (remainingTimes) => {
fn()
.then(resolve)
.catch((error) => {
if (remainingTimes > 0) {
setTimeout(() => {
attempt(remainingTimes - 1);
}, delay);
} else {
reject(error);
}
});
};
attempt(times);
});
}
```
这个函数接受三个参数:`fn`是要执行的函数,`times`是尝试的次数,`delay`是延迟的时间(以毫秒为单位)。它返回一个Promise,要么成功地解析出结果,要么在尝试完指定次数后被拒绝。
你可以使用它来尝试执行一个可能会失败的异步操作,例如:
```javascript
function fetchData() {
return new Promise((resolve, reject) => {
// 模拟一个异步操作,这里使用setTimeout来延迟1秒
setTimeout(() => {
const random = Math.random();
if (random < 0.8) {
resolve('Data fetched successfully');
} else {
reject('Error: Failed to fetch data');
}
}, 1000);
});
}
retry(fetchData, 3, 1000)
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
```
在上面的示例中,`fetchData`函数模拟了一个可能失败的异步操作。我们使用`retry`函数来尝试执行`fetchData`函数,最多尝试3次,每次间隔1秒。如果在3次尝试后仍然失败,将会拒绝Promise并打印错误信息。如果成功地获取到数据,将会解析Promise并打印数据。
阅读全文
相关推荐
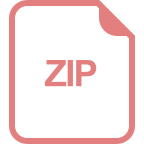
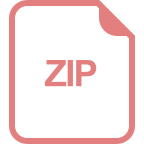
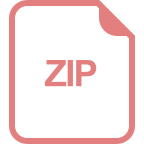
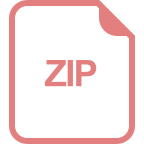
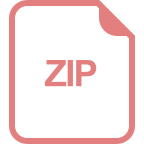
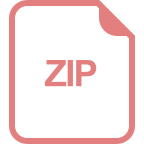
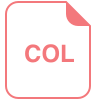










