我想用python批量处理文件夹中的图片,删除文件夹下小于指定大小的图片
时间: 2024-09-26 20:14:18 浏览: 36
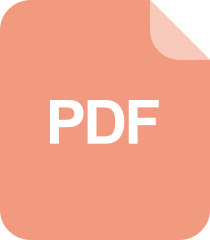
Python实现图片批量加入水印代码实例
在Python中,你可以使用`os`, `shutil`, 和 `PIL`(Pillow库,用于图像操作)来批量处理文件夹中的图片并删除尺寸不符合条件的。以下是一个简单的步骤:
首先,你需要安装Pillow库,如果还没有安装,可以使用pip来安装:
```bash
pip install pillow
```
然后,编写一个脚本,例如:
```python
import os
from PIL import Image
# 定义目标文件夹路径和最小允许的图片尺寸(这里以像素为单位)
folder_path = 'your_folder_path'
min_size = (500, 500) # 高度和宽度
def check_image_size(file_path):
try:
with Image.open(file_path) as img:
width, height = img.size
if width >= min_size[0] and height >= min_size[1]:
return True
else:
print(f"Image {file_path} is too small, size: {img.size}, removing...")
except Exception as e:
print(f"Error processing {file_path}: {e}")
# 如果图片尺寸不够大,删除它
shutil.rmtree(os.path.dirname(file_path), ignore_errors=True)
return False
# 遍历文件夹及其所有子目录中的图片
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith(('.jpg', '.jpeg', '.png', '.gif')): # 只处理常见的图片格式
file_path = os.path.join(root, file)
if not check_image_size(file_path):
continue # 文件已删除,跳过
```
这个脚本会检查文件夹下的每个图片,如果尺寸小于指定大小,则删除该图片。注意,删除操作是立即执行的,所以请谨慎设置`min_size`。
阅读全文
相关推荐
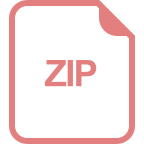
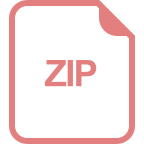


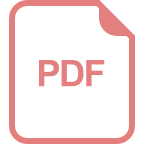
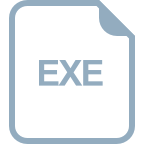

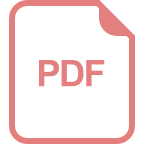
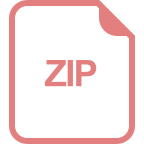
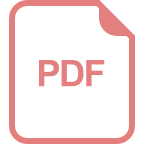
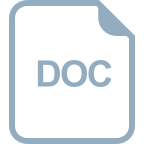
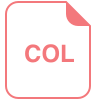
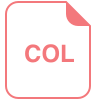
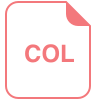



