vector<int>
时间: 2024-06-02 20:03:42 浏览: 78
vector<int>是C++标准库中的容器类,用于存储一组整数类型的元素。它提供了动态数组的功能,可以根据需要自动调整大小。以下是vector<int>的一些特点和常用操作:
1. 特点:
- 动态大小:vector<int>可以根据需要自动调整大小,可以动态地添加或删除元素。
- 连续存储:vector<int>的元素在内存中是连续存储的,可以索引快速访元素。
- 支持随机访问:可以通过下访问元素,时间复杂度为O(1)。
- 自动管理内存:vector<int>会自动管理内存,当元素数量超过当前容量时,会重新分配更大的内存空间。
2. 常用操作:
- 添加元素:可以使用push_back()函数在vector<int>的末尾添加一个元素。
- 删除元素:可以使用pop_back()函数删除vector<int>的末尾元素。
- 访问元素:可以使用下标运算符[]或at()函数来访问指定位置的元素。
- 获取大小:可以使用size()函数获取vector<int>中元素的数量。
- 判断是否为空:可以使用empty()函数判断vector<int>是否为空。
- 清空容器:可以使用clear()函数清空vector<int>中的所有元素。
相关问题
vector<vector<int>>
vector< vector<int> > 是一个容器,其中嵌套了另一个容器。外层容器的元素类型是 vector<int>,内层容器的元素类型是 int。你可以通过以下几个步骤来使用 vector< vector<int> >:
1. 定义和长度:
- 正确的定义方式:vector< vector<int> > A;(在尖括号前加上空格)
- 获取 vector< vector<int> > A 中的外层容器元素个数:int len = A.size();
- 获取 vector< vector<int> > A 中第 i 个外层容器的长度:int len = A[i].size();
2. 访问某个元素:
- 在使用 vector 容器之前,需要加上 <vector> 头文件:#include <vector>
- vector 属于 std 命名空间的内容,因此需要通过命名限定:using std::vector;(也可以直接使用全局的命名空间方式:using namespace std;)
- 使用 vector 的成员函数来访问元素...
例如,如果想定义 A = [[0,1,2],[3,4,5]],可以按如下步骤:
```cpp
vector< vector<int> > A; // 大容器
// A.push_back 里必须是 vector
vector<int> B; // 小容器
B.push_back(0);
B.push_back(1);
B.push_back(2);
A.push_back(B); // 小容器放入大容器
B.clear(); // 清空小容器元素
B.push_back(3);
B.push_back(4);
B.push_back(5);
A.push_back(B);
```
范例中还提供了一个案例,可以参考如下代码实现键盘输入 n 维矩阵,转化为二维向量:
```cpp
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector< vector<int> > v;
vector<int> temp;
int n, i, j, num;
cout << "input the dimension:";
cin >> n;
cout << "请输入" << n << "*" << n << "的矩阵" << endl;
// 输入元素
for (i = 0; i < n; i++) {
temp.clear(); // 清空 temp 内元素
for (j = 0; j < n; j++) {
cin >> num;
temp.push_back(num);
}
v.push_back(temp);
}
cout << "<----- 遍历输出所有元素 ----->" << endl;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
cout << v[i][j] << " ";
}
cout << endl;
}
return 0;
}
```
vector<vector<int>>
This is a two-dimensional vector in C++. It is a vector that contains other vectors as its elements. Each element vector can have a different size and can contain any type of data, including integers in this case.
Here's an example of how to declare and initialize a vector<vector<int>>:
```c++
#include <vector>
using namespace std;
int main() {
// Declare and initialize a 2D vector with 3 rows and 4 columns
vector<vector<int>> matrix = { {1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12} };
// Accessing an element in the matrix
int element = matrix[1][2]; // Returns 7
}
```
In this example, `matrix` is a 2D vector with 3 rows and 4 columns. The first row contains the integers 1, 2, 3, and 4, the second row contains 5, 6, 7, and 8, and the third row contains 9, 10, 11, and 12. To access an element in the matrix, we use two square brackets - the first one specifies the row index and the second one specifies the column index. In this case, `matrix[1][2]` returns the element in the second row and third column, which is 7.
阅读全文
相关推荐
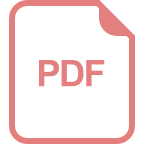








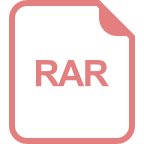