html星空粒子特效代码
时间: 2023-08-26 18:11:29 浏览: 157
以下是一个简单的HTML星空粒子特效代码:
```html
<!DOCTYPE html>
<html>
<head>
<style>
body {
margin: 0;
overflow: hidden;
}
canvas {
display: block;
}
</style>
</head>
<body>
<canvas id="starfield"></canvas>
<script>
// 创建画布
var canvas = document.getElementById("starfield");
var ctx = canvas.getContext("2d");
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 创建星星数组
var stars = [];
var numStars = 100;
// 初始化星星
for (var i = 0; i < numStars; i++) {
stars[i] = {
x: Math.random() * canvas.width,
y: Math.random() * canvas.height,
radius: Math.random() * 1.5 + 1,
vx: Math.floor(Math.random() * 3) - 1.5,
vy: Math.floor(Math.random() * 3) - 1.5
};
}
// 绘制星星
function drawStars() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = "white";
for (var i = 0; i < numStars; i++) {
var star = stars[i];
ctx.beginPath();
ctx.arc(star.x, star.y, star.radius, 0, Math.PI * 2, false);
ctx.fill();
}
}
// 更新星星位置
function updateStars() {
for (var i = 0; i < numStars; i++) {
var star = stars[i];
// 更新位置
star.x += star.vx;
star.y += star.vy;
// 边界检测
if (star.x < 0 || star.x > canvas.width) {
star.vx = -star.vx;
}
if (star.y < 0 || star.y > canvas.height) {
star.vy = -star.vy;
}
}
}
// 动画循环
function animate() {
drawStars();
updateStars();
requestAnimationFrame(animate);
}
// 开始动画
animate();
</script>
</body>
</html>
```
这段代码使用HTML5的canvas元素绘制了一个星空粒子特效。它创建了一个画布,然后在画布上绘制了一定数量的随机大小和位置的白色圆形来表示星星。每个星星都有一个随机的速度,它们会在画布上移动,并在达到边界时反弹。通过不断更新星星的位置并重新绘制它们,实现了一个看起来像星空的动态效果。你可以将该代码复制粘贴到一个HTML文件中,然后在浏览器中打开该文件来查看效果。
阅读全文
相关推荐
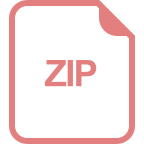
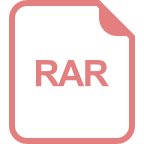
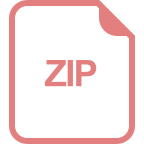
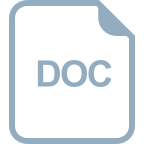
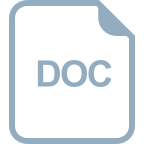
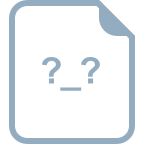
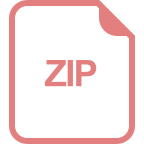
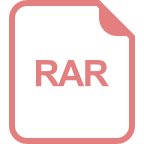
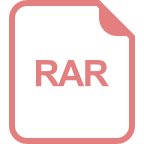
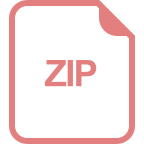
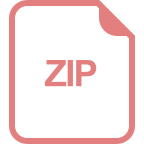
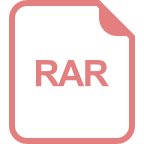
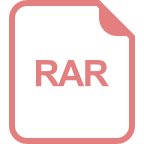
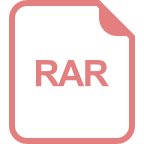
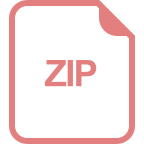
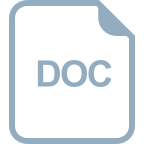