C# 条件可选 联表查询 代码
时间: 2024-09-25 14:13:19 浏览: 52
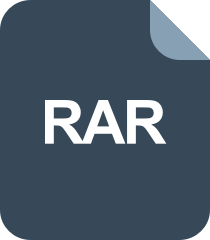
C#23种设计模式_示例源代码及PDF

在C#中,如果你想要执行条件可选的联表查询(也称为JOIN操作),特别是在LINQ(Language Integrated Query)上下文中,你可以使用`Join`或`GroupJoin`方法。假设你有两个实体类,比如`Customer`和`Order`,并且你想找出每个客户是否有订单,你可以这样做:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
}
public class Order
{
public int Id { get; set; }
public int CustomerId { get; set; }
public DateTime Date { get; set; }
}
class Program
{
static void Main(string[] args)
{
// 假设这是两个实体的示例数据
List<Customer> customers = new List<Customer>
{
new Customer { Id = 1, Name = "Alice" },
new Customer { Id = 2, Name = "Bob" },
new Customer { Id = 3, Name = "Charlie" }
};
List<Order> orders = new List<Order>
{
new Order { Id = 1, CustomerId = 1, Date = DateTime.Now },
new Order { Id = 2, CustomerId = 2, Date = DateTime.Now.AddDays(-1) },
};
// 使用 LINQ 进行联表查询
var result = from customer in customers
join order in orders on customer.Id equals order.CustomerId into customerOrders
from orderInCustomer in customerOrders.DefaultIfEmpty() // 条件可选
select new
{
Customer = customer,
HasOrder = orderInCustomer != null,
LatestOrderDate = orderInCustomer?.Date // 如果有订单,则获取日期
};
foreach (var item in result)
{
Console.WriteLine($"Customer: {item.Customer.Name}, Has Order: {item.HasOrder}, Latest Order Date: {item.LatestOrderDate}");
}
// 或者使用扩展方法版本
result = customers.Join(orders,
c => c.Id,
o => o.CustomerId,
(c, o) => new
{
Customer = c,
HasOrder = o != null,
LatestOrderDate = o.Date ?? default(DateTime)
});
// ...继续处理结果
}
}
```
在这个例子中,`DefaultIfEmpty()` 方法确保即使某个客户没有订单,也会返回一个空的 `Order` 对象,表示该条件为假。`??` 运算符用于安全地提供默认值。
阅读全文
相关推荐
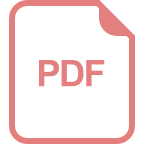
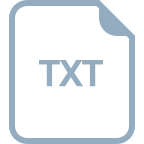

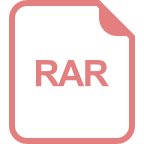
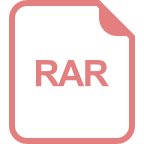
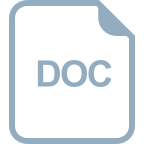
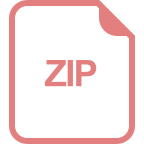
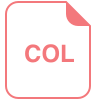
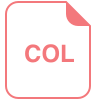
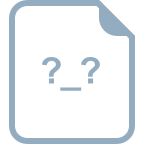
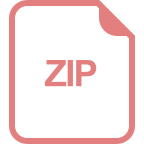