使用C语言编写打印单链表中所有数据的函数。
时间: 2024-10-13 20:09:21 浏览: 29
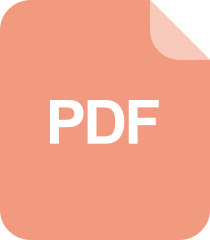
C语言实现学生信息管理系统(单链表)
在C语言中,你可以创建一个函数来遍历并打印单链表的所有元素。首先,你需要定义一个结构体表示链表节点,然后定义一个指向头节点的指针。这里是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data; // 节点的数据域
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL;
}
return newNode;
}
// 打印链表所有数据的函数
void printLinkedList(Node* head) {
Node* current = head; // 初始化当前节点为头节点
while (current != NULL) { // 当还有节点时循环
printf("%d ", current->data); // 打印当前节点的数据
current = current->next; // 移动到下一个节点
}
printf("\n"); // 结束打印后换行
}
// 示例链表的主函数
int main() {
Node* listHead = createNode(1);
listHead->next = createNode(2);
listHead->next->next = createNode(3); // 假设这是一个有三个元素的链表
printLinkedList(listHead); // 调用打印函数
return 0;
}
```
在这个示例中,`printLinkedList`函数接受链表的头节点作为参数,并通过`while`循环依次访问每个节点并打印其数据。
阅读全文
相关推荐
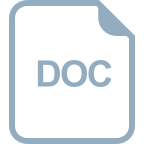
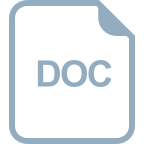

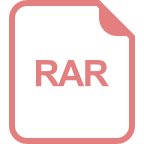













