c语言创建有序单链表
时间: 2024-10-08 16:16:10 浏览: 39
在C语言中,创建有序单链表通常涉及到节点结构的定义、链表的初始化以及插入元素保持顺序的操作。下面是一个简单的步骤概述:
1. **定义节点结构**:
```c
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. **初始化链表**:
由于C语言默认不会自动排序元素,我们需要手动处理插入操作。开始时,链表的头节点通常是`NULL`。
```c
Node* sortedList = NULL;
```
3. **插入元素**:
插入函数需要判断新元素应该插入的位置以保持链表有序。例如,可以编写一个`insertSorted`函数:
```c
void insertSorted(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
if (*head == NULL || value < (*head)->data) {
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data > value) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
```
4. **遍历链表示例**:
如果需要打印链表内容,可以用一个循环遍历节点:
```c
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
```
阅读全文
相关推荐
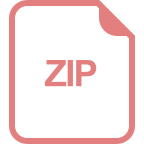
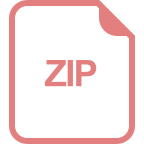


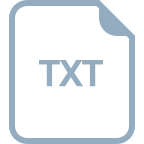
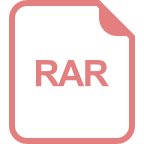
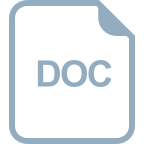
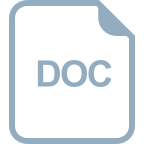
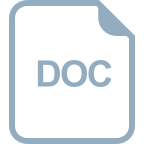








