封装点Point类和线段Line类。点有x,y属性线段有起点和终点主程序中创建两个点用这两个点创建一条线段输出线段的长度。要求封装类完整结果输出正确
时间: 2024-09-21 13:12:34 浏览: 75
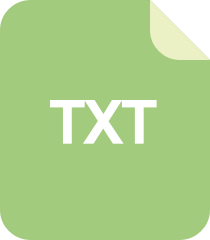
声明一个类Point,然后利用它声明一个组合类Line,实现由用户输入两点确定直线的长度和斜率,编写程序实现测试

首先,我们来创建两个封装类:`Point` 和 `Line`。
```java
// 点类 Point
public class Point {
private double x;
private double y;
// 构造函数
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// 获取和设置坐标值
public double getX() { return x; }
public void setX(double newX) { x = newX; }
public double getY() { return y; }
public void setY(double newY) { y = newY; }
// 计算两点之间的距离,用于后续计算线段长度
public double distance(Point otherPoint) {
double dx = otherPoint.getX() - x;
double dy = otherPoint.getY() - y;
return Math.sqrt(dx * dx + dy * dy);
}
}
// 线段类 Line
public class Line {
private Point start;
private Point end;
// 构造函数,接受两个点作为线段的起点和终点
public Line(Point start, Point end) {
this.start = start;
this.end = end;
}
// 设置和获取起点和终点
public Point getStart() { return start; }
public void setStart(Point newStart) { start = newStart; }
public Point getEnd() { return end; }
public void setEnd(Point newEnd) { end = newEnd; }
// 计算线段长度
public double length() {
return start.distance(end);
}
}
// 主程序
public class Main {
public static void main(String[] args) {
// 创建两个点
Point p1 = new Point(0, 0);
Point p2 = new Point(3, 4);
// 使用这两个点创建线段
Line line = new Line(p1, p2);
// 输出线段的长度
System.out.println("线段长度: " + line.length());
}
}
```
在这个例子中,`Point` 类封装了点的位置信息,并提供了一个计算两点间距离的方法。`Line` 类则封装了线段的起始和结束点,以及计算线段长度的功能。在主程序中,我们创建了两个点并使用它们构造了一条线段,最后输出了线段的长度。运行这个程序,将得到线段的正确长度。
阅读全文
相关推荐
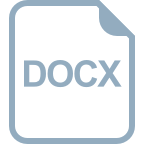
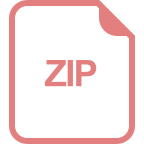





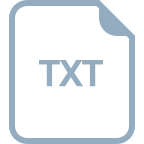
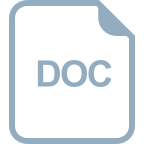
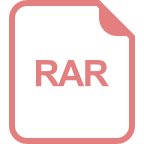
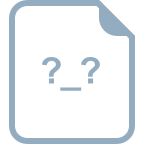
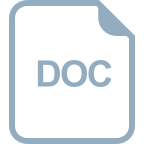
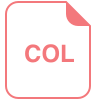
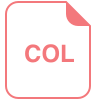


