/封装点 Point 类和线段 Line 类。点有x.y属性;线段有起点和终点。主程序中创建两不点,用这两个点创建一条线段,输出线段的长度。要求:类封装完整,结果输出正确。
时间: 2024-09-22 17:07:19 浏览: 18
在面向对象编程中,我们可以创建两个类来分别表示点和线段的概念。首先,我们定义一个`Point`类,它包含两个私有成员变量`x`和`y`,代表点的坐标,并提供公有的getters和setters来访问这些值。
```java
public class Point {
private double x;
private double y;
// 构造函数
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// Getter and Setter methods
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
}
```
接着,我们创建一个`Line`类,这个类有两个`Point`类型的属性,表示线段的起点和终点。线段类会有一个计算长度的方法,使用两点间距离公式来获取长度:
```java
public class Line {
private Point start;
private Point end;
// 构造函数,接收两个点作为参数
public Line(Point start, Point end) {
this.start = start;
this.end = end;
}
// 计算并返回线段长度
public double getLength() {
double deltaX = end.getX() - start.getX();
double deltaY = end.getY() - start.getY();
return Math.sqrt(deltaX * deltaX + deltaY * deltaY);
}
}
```
最后,在主程序中,你可以这样创建点和线段,并打印出线段的长度:
```java
public static void main(String[] args) {
Point p1 = new Point(0, 0); // 创建第一个点
Point p2 = new Point(3, 4); // 创建第二个点
Line line = new Line(p1, p2); // 使用点创建线段
double length = line.getLength(); // 获取线段长度
System.out.println("线段长度: " + length);
}
```
相关推荐
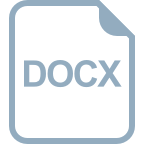
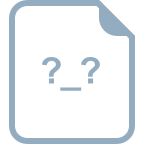
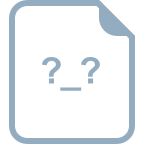














