数据结构链式主串求子串
时间: 2023-11-26 10:47:42 浏览: 102
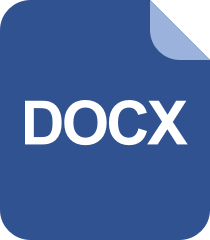
数据结构-c语言-带main函数-串6-求子串-根据标准库函数substr()和定义求子串函数的两种方法。
链式存储结构是一种常见的数据结构存储方式,它通过指针将一组零散的内存块串联起来,形成一个完整的数据结构。链式存储结构可以用来存储任意长度的数据,包括字符串。下面是链式存储结构实现主串求子串的方法:
1. 定义链式存储结构
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
```
2. 初始化链表
```python
def initList(self, data):
self.head = Node(data[0])
p = self.head
for i in data[1:]:
p.next = Node(i)
p = p.next
```
3. 打印链表
```python
def printList(self):
p = self.head
while p:
print(p.data, end='')
p = p.next
print()
```
4. 求链表长度
```python
def getLength(self):
p = self.head
length = 0
while p:
length += 1
p = p.next
return length
```
5. 求子串
```python
def getSubList(self, start, end):
if start < 1 or end > self.getLength() or start > end:
return None
p = self.head
subList = LinkedList()
for i in range(1, end+1):
if i >= start:
subList.addNode(p.data)
p = p.next
return subList
```
6. 示例
```python
# 初始化链表
linkedList = LinkedList()
linkedList.initList('abcdefg')
# 打印链表
linkedList.printList() # 输出:abcdefg
# 求子串
subList = linkedList.getSubList(2, 5)
subList.printList() # 输出:bcde
```
阅读全文
相关推荐
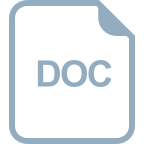
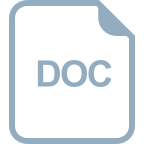
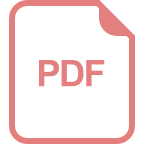
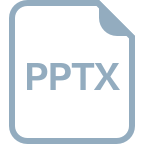
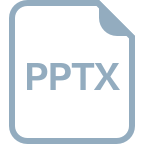
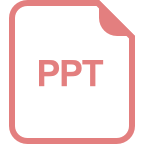
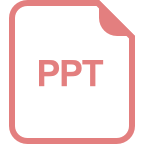
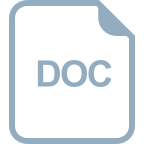
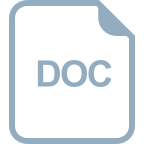
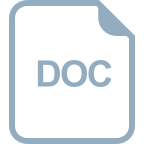
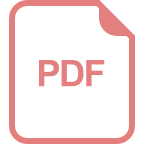
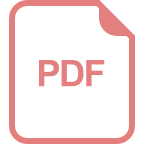
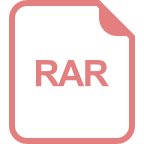
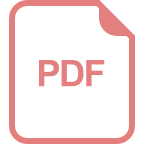
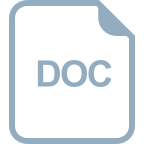