springboot 集成elasticsearch 示例
时间: 2023-07-30 18:04:03 浏览: 67
以下是一个简单的示例,展示如何在Spring Boot中集成Elasticsearch:
1. 添加依赖:在你的Spring Boot项目的pom.xml文件中,添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
```
2. 配置连接信息:在application.properties(或application.yml)文件中,配置Elasticsearch的连接信息,例如:
```properties
spring.data.elasticsearch.cluster-nodes=localhost:9200
```
3. 创建实体类:创建一个与Elasticsearch索引对应的实体类,可以使用`@Document`注解指定索引名称和类型,以及使用`@Field`注解指定字段的映射关系。例如:
```java
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
@Document(indexName = "my_index", type = "my_type")
public class MyEntity {
@Id
private String id;
@Field(type = FieldType.Text)
private String name;
// getter/setter
}
```
4. 创建Repository接口:创建一个继承自`ElasticsearchRepository`的接口,用于对Elasticsearch进行操作。例如:
```java
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
public interface MyEntityRepository extends ElasticsearchRepository<MyEntity, String> {
}
```
5. 使用Repository进行操作:在你的服务类或控制器中,注入`MyEntityRepository`并使用它进行操作。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class MyService {
@Autowired
private MyEntityRepository repository;
public void save(MyEntity entity) {
repository.save(entity);
}
public List<MyEntity> search(String keyword) {
return repository.findByName(keyword);
}
}
```
这样,你就完成了Spring Boot与Elasticsearch的集成。你可以通过调用`save`方法将实体对象保存到Elasticsearch中,通过调用`search`方法进行搜索操作。
请注意,这只是一个简单的示例,你可以根据自己的需求进行更复杂的操作和配置。更多关于Spring Data Elasticsearch的使用方法,请参考官方文档。
相关推荐
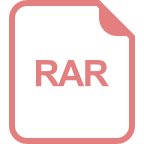
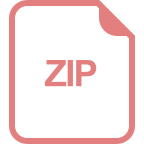
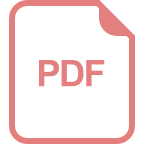














