如何使用分治法来求解并可视化二维空间中指定数量点的凸包?例如,生成一个含有N个随机点的集合,并展示其对应的凸包图形,类似于示例图(b)。请提供详细步骤和代码实现过程。
时间: 2024-12-28 22:32:03 浏览: 7
分治法用于求解二维空间中点集的凸包通常是通过坐标轴覆盖算法,如“gift wrapping”算法或“ Graham Scan”。以下是详细的步骤:
1. **初始化**:首先,选择任意一个未排序的点作为起点`p`,通常选择第一个点。
2. **构建堆栈**:将剩下的点按照逆时针顺序加入一个最小堆栈,同时检查是否形成了顺时针方向的环。如果有,说明`p`是凸包的一个顶点,然后跳过这个循环。
3. **遍历堆栈**:每次从堆栈顶部取出一个点`q`,并与当前已知的凸包边相连。如果新的边连接到堆栈顶部`q`和下一个点`r`形成更大的角度,则更新凸包边为`pq`,并将`r`替换到堆栈上。
4. **继续迭代**:重复步骤3,直到堆栈为空。
5. **凸包结果**:最后的凸包就是由已处理过的点组成的多边形。
以下是使用Python和matplotlib库简单实现上述过程的一个示例:
```python
import matplotlib.pyplot as plt
import random
from heapq import heappush, heappop
def get_convex_hull(points):
if len(points) < 3:
return points
# Choose an arbitrary point as start
p = points[0]
hull = [p]
# Build a min heap with the rest of the points
hq = [(x - p[0], y - p[1]) for x, y in points[1:]]
heappush(hq, (random.random(), random.random())) # Randomize to avoid ties
while hq:
q = heappop(hq)
while hq and cross(p[-1] - q, q - hq[0]) <= 0:
heappop(hq)
p.append(q)
hq.append(p[-1])
return p[:-1] # Remove the last duplicate point
# Generate N random points
N = 10
points = [(random.random(), random.random()) for _ in range(N)]
# Sort points and find convex hull
sorted_points = sorted(points, key=lambda pt: pt[0])
convex_hull = get_convex_hull(sorted_points)
# Plot points and convex hull
plt.scatter(*zip(*points), color='blue', marker='o')
plt.plot(*zip(*convex_hull), color='red', linewidth=2)
plt.title(f"Convex Hull of {N} Random Points")
plt.show()
阅读全文
相关推荐











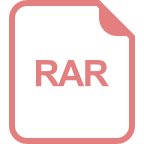

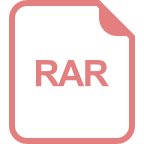

