unity OnPointerEnter
时间: 2024-12-01 19:08:43 浏览: 14
在Unity引擎中,`OnPointerEnter` 是一个预设的Unity事件,它会在游戏对象的鼠标指针进入其碰撞区域时触发。这个方法通常用于处理交互式UI元素,例如悬浮菜单、按钮等,当玩家的鼠标移到这些元素上时,会执行指定的脚本代码。
在这个事件里,你可以编写C#代码来响应鼠标进入的行为,比如改变UI状态、播放动画、显示提示信息等。这个方法接受一个参数 `PointerEventData eventData`,包含了关于这次鼠标事件的详细信息,如鼠标位置、触摸板ID等。
示例代码:
```csharp
public class MyObject : MonoBehaviour
{
void OnPointerEnter(PointerEventData eventData)
{
Debug.Log("Mouse entered the object.");
// 更改物体颜色或其他UI反馈
GetComponent<Renderer>().material.color = Color.green;
}
}
```
相关问题
Unity EventTriggerListener
Unity EventTriggerListener is a script that allows you to easily add event listeners to Unity's Event Trigger component. The Event Trigger component is a component that allows you to specify a set of events to listen to on a GameObject, such as mouse clicks, mouse over, or drag events.
With the EventTriggerListener script, you can easily add listeners to these events without having to write any code. Simply attach the script to the GameObject with the Event Trigger component, and then add the desired listeners in the Inspector.
The script provides a simple interface for adding listeners to the various events supported by the Event Trigger component. You can add listeners for events such as OnPointerClick, OnPointerEnter, OnPointerExit, OnDrag, and many more.
The EventTriggerListener script is useful for adding interactivity to your Unity projects without having to write a lot of code. It allows you to easily respond to user input and create engaging experiences for your users.
unity扇形按钮
你可以使用Unity的UGUI系统来创建扇形按钮。以下是一个简单的示例:
1.创建一个新的Canvas对象,然后将其RectTransform的锚点设置为(0, 0)并将其拉伸到全屏幕大小。
2.在Canvas下创建一个新的Empty GameObject,并将其命名为“RadialMenu”。
3.将RadialMenu的RectTransform的锚点设置为(0.5, 0.5),并将其位置设置为Canvas的中心。
4.为RadialMenu添加一个Image组件,然后将其颜色设置为透明。
5.创建一个新的Empty GameObject,并将其命名为“ButtonTemplate”。
6.将ButtonTemplate放置在RadialMenu内部,并将其RectTransform的位置设置为(0, 0)。
7.为ButtonTemplate添加一个Image组件,并设置其颜色为白色。
8.为ButtonTemplate添加一个Button组件。
9.创建一个新的Empty GameObject,并将其命名为“Icon”。
10.将Icon放置在ButtonTemplate内部,并将其RectTransform的位置设置为(0, 0)。
11.为Icon添加一个Image组件,并设置其图片为你想要的图标。
12.为Icon添加一个Text组件,并设置其内容为你想要的文本。
13.创建一个新的C#脚本,并将其命名为“RadialMenuButton”。
14.将RadialMenuButton脚本附加到ButtonTemplate上,并将其Icon和Text属性设置为Icon和Text游戏对象。
15.在RadialMenuButton脚本中添加以下代码:
```
using UnityEngine;
using UnityEngine.UI;
public class RadialMenuButton : MonoBehaviour
{
public GameObject Icon;
public GameObject Text;
[HideInInspector]
public float Angle;
private Button button;
void Awake()
{
button = GetComponent<Button>();
}
void Update()
{
transform.rotation = Quaternion.Euler(0, 0, Angle);
}
}
```
这个脚本将Icon和Text属性公开给RadialMenuButton组件,并在Update方法中将按钮旋转到正确的角度。
16.回到RadialMenu GameObject,创建一个新的C#脚本,并将其命名为“RadialMenu”。
17.将RadialMenu脚本附加到RadialMenu上,并添加以下代码:
```
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.EventSystems;
using System.Collections.Generic;
public class RadialMenu : MonoBehaviour, IPointerEnterHandler, IPointerExitHandler
{
public GameObject ButtonTemplate;
public int ButtonCount;
public float ButtonSize;
public float ButtonDistance;
public float OpenTime;
public float CloseTime;
public bool IsOpen;
private List<RadialMenuButton> buttons = new List<RadialMenuButton>();
private float angleOffset;
private bool isPointerOver;
void Start()
{
angleOffset = 360f / ButtonCount;
for (int i = 0; i < ButtonCount; i++)
{
GameObject button = Instantiate(ButtonTemplate);
button.transform.SetParent(transform, false);
button.transform.localPosition = Vector3.zero;
button.transform.localScale = Vector3.one;
button.SetActive(true);
RadialMenuButton radialButton = button.GetComponent<RadialMenuButton>();
radialButton.Angle = angleOffset * i;
buttons.Add(radialButton);
}
ButtonTemplate.SetActive(false);
}
void Update()
{
if (isPointerOver)
{
if (!IsOpen)
{
IsOpen = true;
StartCoroutine(Open());
}
}
else
{
if (IsOpen)
{
IsOpen = false;
StartCoroutine(Close());
}
}
}
IEnumerator<WaitForSeconds> Open()
{
for (int i = 0; i < ButtonCount; i++)
{
RadialMenuButton button = buttons[i];
float x = Mathf.Sin(Mathf.Deg2Rad * button.Angle) * ButtonDistance;
float y = Mathf.Cos(Mathf.Deg2Rad * button.Angle) * ButtonDistance;
button.transform.localPosition = new Vector3(x, y, 0f) * ButtonSize;
yield return new WaitForSeconds(OpenTime);
}
}
IEnumerator<WaitForSeconds> Close()
{
for (int i = 0; i < ButtonCount; i++)
{
RadialMenuButton button = buttons[i];
button.transform.localPosition = Vector3.zero;
yield return new WaitForSeconds(CloseTime);
}
}
public void OnPointerEnter(PointerEventData eventData)
{
isPointerOver = true;
}
public void OnPointerExit(PointerEventData eventData)
{
isPointerOver = false;
}
}
```
这个脚本将RadialMenu的按钮动画化,并在鼠标悬停时打开菜单。
18.在RadialMenu上添加RadialMenu脚本,并将ButtonTemplate属性设置为你刚刚创建的ButtonTemplate对象。
19.调整RadialMenu的属性,例如ButtonCount、ButtonSize和ButtonDistance,以适应你的需求。
20.按下Play按钮来测试你的扇形按钮。
阅读全文
相关推荐
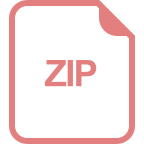
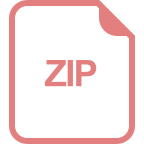
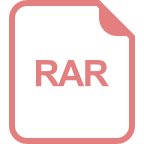













