python实现IIR带通滤波器
时间: 2024-03-15 10:39:10 浏览: 18
Python可以使用信号处理库SciPy来实现IIR(Infinite Impulse Response)带通滤波器。下面是一个简单的示例代码:
```python
import numpy as np
from scipy.signal import butter, filtfilt
def butter_bandpass(lowcut, highcut, fs, order=5):
nyquist = 0.5 * fs
low = lowcut / nyquist
high = highcut / nyquist
b, a = butter(order, [low, high], btype='band')
return b, a
def butter_bandpass_filter(data, lowcut, highcut, fs, order=5):
b, a = butter_bandpass(lowcut, highcut, fs, order=order)
y = filtfilt(b, a, data)
return y
# 示例使用
fs = 1000 # 采样频率
lowcut = 10 # 带通滤波器的低频截止频率
highcut = 100 # 带通滤波器的高频截止频率
# 生成示例信号
t = np.linspace(0, 1, fs, endpoint=False)
data = np.sin(2 * np.pi * 30 * t) + np.sin(2 * np.pi * 150 * t) + 0.5 * np.random.randn(t.size)
# 应用带通滤波器
filtered_data = butter_bandpass_filter(data, lowcut, highcut, fs, order=6)
```
在上面的示例中,`butter_bandpass`函数用于设计IIR带通滤波器的传递函数,`butter_bandpass_filter`函数用于应用滤波器到输入信号上。你可以根据需要调整截止频率、采样频率和滤波器阶数来适应你的应用。
相关推荐
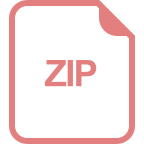














