条带池化层pytorch实现
时间: 2024-04-18 17:22:45 浏览: 14
条带池化层(strip pooling layer)是一种用于文本分类任务的池化操作,它可以提取文本中的关键信息。在PyTorch中,可以通过自定义模块来实现条带池化层。
下面是一个简单的条带池化层的PyTorch实现示例:
```python
import torch
import torch.nn as nn
class StripPoolingLayer(nn.Module):
def __init__(self, strip_size):
super(StripPoolingLayer, self).__init__()
self.strip_size = strip_size
def forward(self, x):
batch_size, seq_len, embedding_dim = x.size()
num_strips = seq_len // self.strip_size
# Reshape input into strips
x = x.view(batch_size, num_strips, self.strip_size, embedding_dim)
# Apply max pooling along the strip dimension
x, _ = torch.max(x, dim=2)
# Flatten the strips
x = x.view(batch_size, -1)
return x
```
在这个示例中,`StripPoolingLayer`继承自`nn.Module`,并实现了`forward`方法来定义前向传播逻辑。在前向传播过程中,首先将输入张量`x`按照`strip_size`进行切分,然后对每个切分的条带应用最大池化操作,最后将所有条带展平为一个一维张量。
使用该条带池化层的示例代码如下:
```python
# 创建条带池化层实例
strip_pooling = StripPoolingLayer(strip_size=3)
# 输入数据
input_data = torch.tensor([
[[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]],
[[13, 14, 15], [16, 17, 18], [19, 20, 21], [22, 23, 24]]
])
# 前向传播
output = strip_pooling(input_data)
print(output)
```
这里的`input_data`是一个3D张量,表示两个样本的输入文本,每个样本有4个词,每个词的维度是3。经过条带池化层后,输出的张量`output`将是一个2D张量,表示两个样本的条带池化结果。
相关推荐
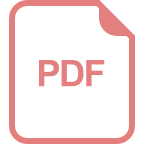
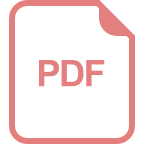
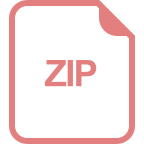














