java多线程读写文件
时间: 2024-12-27 20:20:33 浏览: 4
### Java 多线程 文件读写 实现方法
#### 使用多线程提升文件读写的性能
通过引入多线程技术可以显著提高文件读写操作的效率。当涉及到大量文件或大容量单个文件时,利用并发机制能够充分利用CPU资源,减少I/O等待时间。
对于多线程环境下安全地访问共享资源——即文件对象而言,必须采取适当措施防止竞争条件的发生。这通常意味着要对临界区施加同步控制,比如借助于`ReentrantReadWriteLock`这样的工具类[^2]。
#### 示例代码展示
下面给出了一种基于线程池模式下的异步文件读取方案:
```java
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.AsynchronousFileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.util.concurrent.ExecutionException;
public class AsyncFileReader {
private final Path filePath;
public AsyncFileReader(String fileName){
this.filePath = Paths.get(fileName);
}
/**
* 异步方式打开文件并读取指定长度的内容.
*/
public String readFile(int length) throws IOException, InterruptedException, ExecutionException {
StringBuilder contentBuilder = new StringBuilder();
try (AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(filePath,
StandardOpenOption.READ)) {
ByteBuffer buffer = ByteBuffer.allocate(length);
Integer bytesRead = fileChannel.read(buffer, 0).get();
while(bytesRead != -1 && bytesLeftToRead > 0 ) {
buffer.flip();
byte[] dataBytes = new byte[buffer.remaining()];
buffer.get(dataBytes);
contentBuilder.append(new String(dataBytes));
if (bytesRead < length || bytesLeftToRead <= 0 )
break;
buffer.clear();
bytesRead = fileChannel.read(buffer, bytesRead).get();
}
} catch(IOException e){
throw e;
}
return contentBuilder.toString();
}
}
```
此段程序定义了一个名为 `AsyncFileReader` 的类,它接受一个字符串参数作为待读取的目标文件路径名,并提供了一个公共的方法用于执行实际的读取工作。这里采用了 NIO.2 中新增添的功能组件 —— `AsynchronousFileChannel` 来完成非阻塞式的磁盘 I/O 操作;同时配合使用了 `Future<V>` 接口来获取最终返回的结果集[^5]。
另外一种常见的做法是在主进程中启动若干个工作子进程分别负责不同的任务单元,例如某个特定范围内的记录解析或是某几个分片文档的整体搬运等。这些辅助性的计算节点之间互不干扰却又紧密协作,共同构成了整个应用程序的核心业务逻辑框架结构。
为了确保数据的一致性和完整性,在上述过程中应当考虑加入必要的异常处理流程以及合理的超时设置策略,从而有效规避潜在的风险隐患。
阅读全文
相关推荐
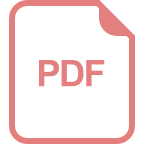
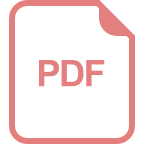















