java多线程 io流导出
时间: 2023-07-06 12:37:28 浏览: 133
如果你需要在Java中使用多线程进行IO操作,可以使用Java的线程池和Java NIO(New IO)库。以下是一个使用线程池和NIO库进行文件导出的示例代码:
```java
import java.io.*;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Exporter {
public static void main(String[] args) {
// 创建线程池
ExecutorService pool = Executors.newFixedThreadPool(10);
// 定义文件路径和文件名
final String filePath = "/path/to/file";
final String fileName = "file.txt";
// 为每个线程分配写入文件的位置
final int blockSize = 1024 * 1024; // 1MB
final long fileSize = 1024 * 1024 * 1024; // 1GB
int blockCount = (int) (fileSize / blockSize);
for (int i = 0; i < blockCount; i++) {
final int blockIndex = i;
pool.execute(new Runnable() {
@Override
public void run() {
try {
// 创建文件通道
RandomAccessFile file = new RandomAccessFile(filePath + fileName, "rw");
FileChannel channel = file.getChannel();
ByteBuffer buffer = ByteBuffer.allocate(blockSize);
// 写入数据
long position = blockIndex * blockSize;
for (int j = 0; j < blockSize; j++) {
buffer.put((byte) (position + j));
}
buffer.flip();
channel.write(buffer, position);
// 关闭文件通道
channel.close();
file.close();
} catch (IOException e) {
e.printStackTrace();
}
}
});
}
// 关闭线程池
pool.shutdown();
}
}
```
在上述代码中,我们使用了一个线程池来管理多个线程,每个线程负责写入文件的一个固定大小的块。我们使用NIO库来读写文件,这样可以提高IO性能。请注意,我们使用的是随机访问文件(RandomAccessFile),这使得我们可以在文件中定位并写入特定位置的字节。在实际应用中,你需要根据实际需求来调整块的大小和线程数。
阅读全文
相关推荐
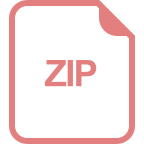




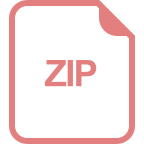
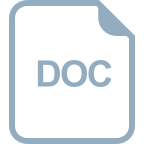
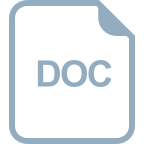
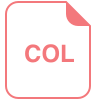
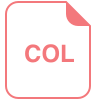
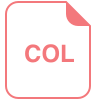
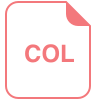



