java 多线程千万级数据导出
时间: 2023-12-03 07:40:47 浏览: 38
以下是Java多线程千万级数据导出的示例代码:
```java
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.metadata.Sheet;
import com.alibaba.excel.support.ExcelTypeEnum;
public class ExcelExportUtil {
/**
* 导出Excel
*
* @param dataList 数据列表
* @param sheetName sheet名称
* @param filePath 文件路径
* @param fileName 文件名
* @param sheetSize 每个sheet中数据的行数
*/
public static void exportExcel(List<List<Object>> dataList, String sheetName, String filePath, String fileName,
int sheetSize) {
// 创建线程池
ExecutorService executorService = Executors.newFixedThreadPool(10);
// 创建ExcelWriter
ExcelWriter excelWriter = new ExcelWriter(new File(filePath + fileName), ExcelTypeEnum.XLSX);
// 计算需要创建的sheet数量
int sheetCount = dataList.size() % sheetSize == 0 ? dataList.size() / sheetSize : dataList.size() / sheetSize + 1;
// 创建计数器
CountDownLatch countDownLatch = new CountDownLatch(sheetCount);
// 创建sheet
for (int i = 0; i < sheetCount; i++) {
// 计算每个sheet中数据的起始位置和结束位置
int startIndex = i * sheetSize;
int endIndex = Math.min(startIndex + sheetSize, dataList.size());
// 创建sheet
Sheet sheet = new Sheet(i + 1, 0);
sheet.setSheetName(sheetName + (i + 1));
// 创建数据列表
List<List<Object>> sheetDataList = new ArrayList<>();
for (int j = startIndex; j < endIndex; j++) {
sheetDataList.add(dataList.get(j));
}
// 将sheet数据提交到线程池中进行处理
executorService.submit(new ExcelExportTask(excelWriter, sheet, sheetDataList, countDownLatch));
}
try {
// 等待所有线程处理完成
countDownLatch.await();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
// 关闭ExcelWriter和线程池
excelWriter.finish();
executorService.shutdown();
}
}
}
```
其中,ExcelExportTask是一个实现了Runnable接口的任务类,用于将数据写入Excel中。具体代码如下:
```java
import java.util.List;
import java.util.concurrent.CountDownLatch;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.metadata.Sheet;
public class ExcelExportTask implements Runnable {
private ExcelWriter excelWriter;
private Sheet sheet;
private List<List<Object>> dataList;
private CountDownLatch countDownLatch;
public ExcelExportTask(ExcelWriter excelWriter, Sheet sheet, List<List<Object>> dataList,
CountDownLatch countDownLatch) {
this.excelWriter = excelWriter;
this.sheet = sheet;
this.dataList = dataList;
this.countDownLatch = countDownLatch;
}
@Override
public void run() {
try {
// 写入数据
excelWriter.write0(dataList, sheet);
} finally {
// 计数器减1
countDownLatch.countDown();
}
}
}
```
使用示例:
```java
import java.util.ArrayList;
import java.util.List;
public class ExcelExportTest {
public static void main(String[] args) {
// 模拟数据
List<List<Object>> dataList = new ArrayList<>();
for (int i = 0; i < 10000000; i++) {
List<Object> row = new ArrayList<>();
row.add("数据" + i);
dataList.add(row);
}
// 导出Excel
ExcelExportUtil.exportExcel(dataList, "Sheet1", "D:/", "data.xlsx", 1000000);
}
}
```
相关推荐
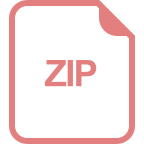














